KY-012 Active Piezo-Buzzer
When this sensor is powered, this active buzzer generates a sound with the frequency of 2.5kHz.
- Arduino
- Raspberry Pi
- Raspberry Pi Pico
- Micro:Bit
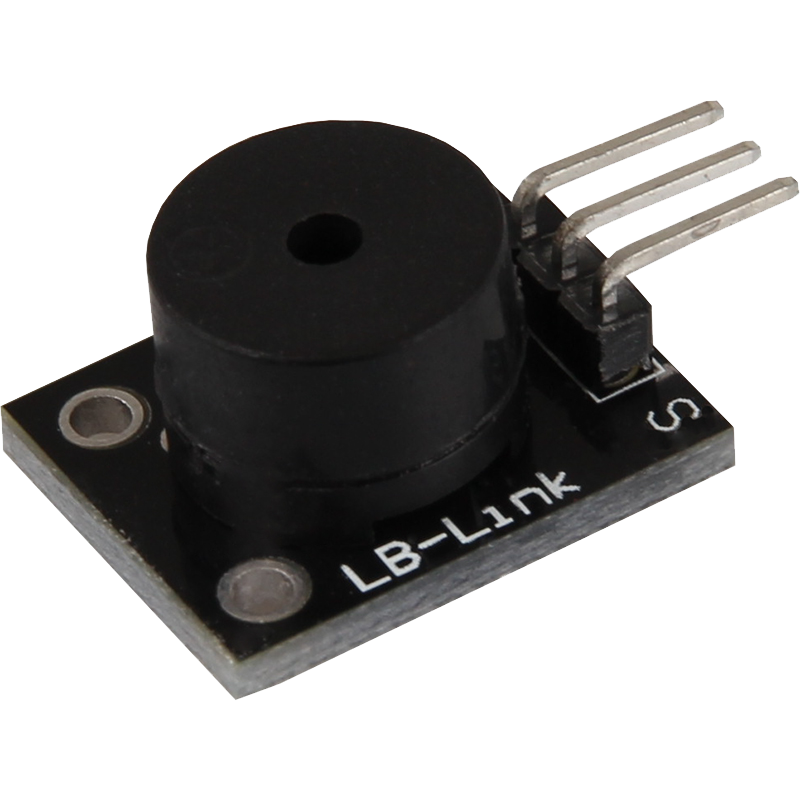
This module contains an active buzzer that immediately generates a sound when it is supplied with voltage. Unlike a passive buzzer, this module does not require an external square-wave voltage to generate a sound. As soon as a voltage of at least 3.3 V is applied to the signal pin, the buzzer automatically generates the necessary square-wave voltage and emits a tone with a frequency of 2.5 kHz.
The buzzer operates in a voltage range of 3.3 V to 5 V and requires a maximum current of 30 mA. It produces a loud sound with a minimum volume of 85 dB, which makes it ideal for applications where a clearly audible acoustic signal is required. This can be useful, for example, in alarm systems, warning systems or as acoustic feedback in various electronic devices.
Technical Data | |
---|---|
Operating voltage | 3,3 V - 5 V |
Maximum current | 30 mA |
Tone frequency | 2,5kHz ± 300 Hz |
Minimum sound output | 85 dB |
Operating temperature | -20 °C - 70 °C |
Storage temperature | -30 °C - 105 °C |
Dimensions | 19 x 15,5 x 11 mm |
Pin assignment
Arduino | Sensor |
---|---|
Pin 13 | Signal |
- | +V |
GND | GND |
Code-Example
This code example shows how the buzzer can be switched on alternately for four seconds and then switched off for two seconds using a definable output pin.
To load the following code example onto your Arduino, we recommend using the Arduino IDE. In the IDE, you can select the appropriate port and board for your device.
Copy the code below into your IDE. To upload the code to your Arduino, simply click on the upload button.
int buzzer = 13;
void setup() {
pinMode(buzzer, OUTPUT); // Initialization output pin for the buzzer
}
// Main program loop
void loop() {
// Buzzer is switched on
digitalWrite(buzzer, HIGH);
// Wait mode for 4 seconds
delay(4000);
// Buzzer is switched off
digitalWrite(buzzer, LOW);
// Waiting for a further two seconds
delay(2000);
}
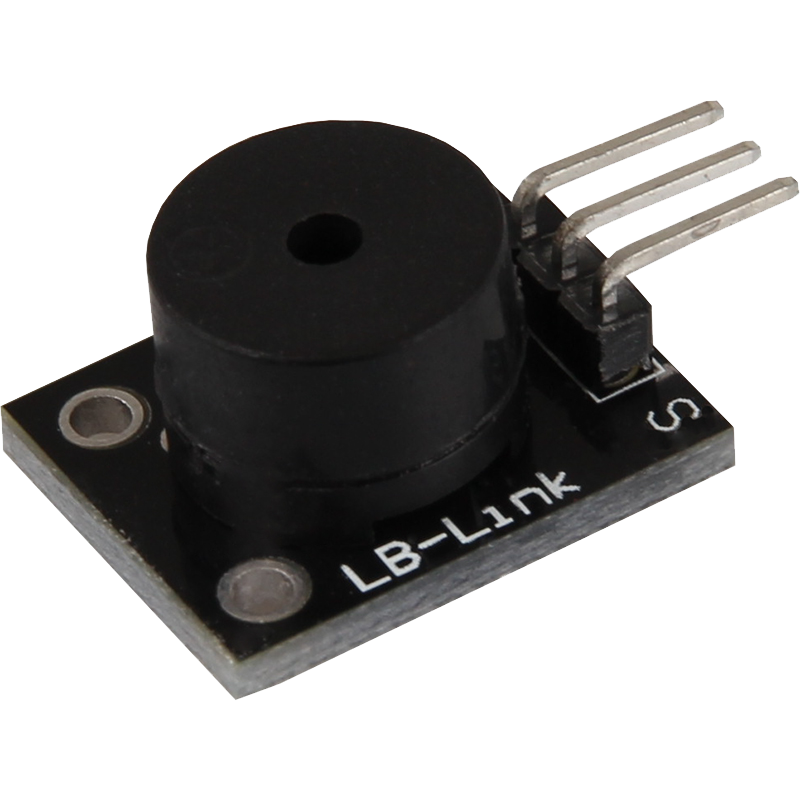
This module contains an active buzzer that immediately generates a sound when it is supplied with voltage. Unlike a passive buzzer, this module does not require an external square-wave voltage to generate a sound. As soon as a voltage of at least 3.3 V is applied to the signal pin, the buzzer automatically generates the necessary square-wave voltage and emits a tone with a frequency of 2.5 kHz.
The buzzer operates in a voltage range of 3.3 V to 5 V and requires a maximum current of 30 mA. It produces a loud sound with a minimum volume of 85 dB, which makes it ideal for applications where a clearly audible acoustic signal is required. This can be useful, for example, in alarm systems, warning systems or as acoustic feedback in various electronic devices.
Technical Data | |
---|---|
Operating voltage | 3,3 V - 5 V |
Maximum current | 30 mA |
Tone frequency | 2,5kHz ± 300 Hz |
Minimum sound output | 85 dB |
Operating temperature | -20 °C - 70 °C |
Storage temperature | -30 °C - 105 °C |
Dimensions | 19 x 15,5 x 11 mm |
Pin assignment
Raspberry Pi | Sensor |
---|---|
GPIO 24 [Pin 18] | Signal |
- | +V |
GND [Pin 6] | GND |
Code-Example
This code example shows how the buzzer can be switched on alternately for four seconds and then switched off for two seconds using a definable output pin.
from gpiozero import Buzzer
import time
# Initialize the Buzzer using the BCM pin number
buzzer = Buzzer(24)
print("Buzzer-Test [press CTRL+C to end the test]")
# Main program loop
try:
while True:
print("Buzzer 4 seconds on")
buzzer.on() # Turn buzzer on
time.sleep(4) # Wait for 4 seconds
print("Buzzer 2 seconds off")
buzzer.off() # Turn buzzer off
time.sleep(2) # Wait for another 2 seconds
# Cleanup after the program has been terminated
except KeyboardInterrupt:
print("Test ended by user")
# gpiozero handles cleanup when the script ends
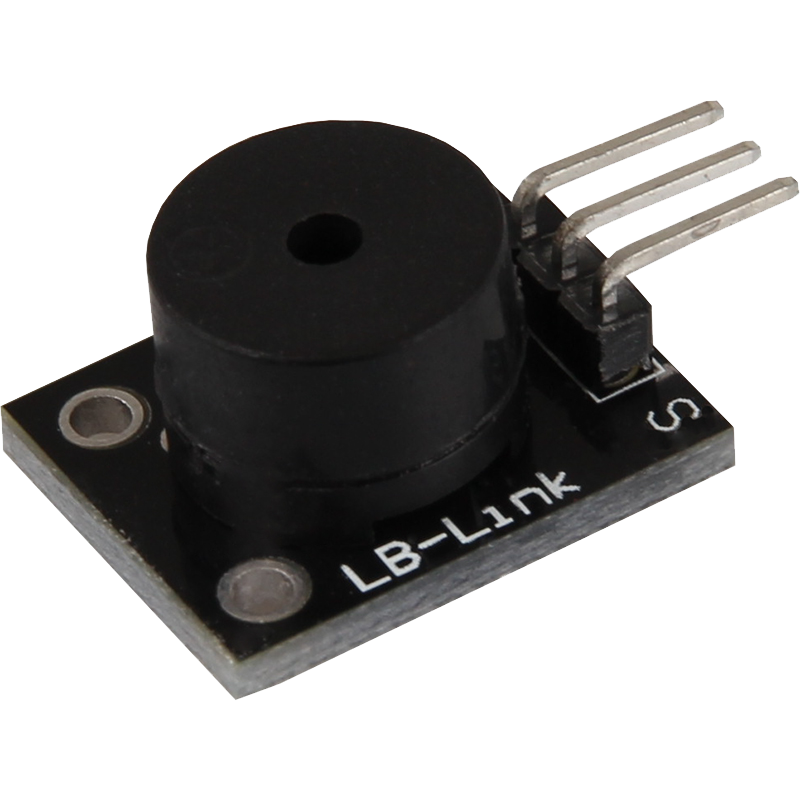
This module contains an active buzzer that immediately generates a sound when it is supplied with voltage. Unlike a passive buzzer, this module does not require an external square-wave voltage to generate a sound. As soon as a voltage of at least 3.3 V is applied to the signal pin, the buzzer automatically generates the necessary square-wave voltage and emits a tone with a frequency of 2.5 kHz.
The buzzer operates in a voltage range of 3.3 V to 5 V and requires a maximum current of 30 mA. It produces a loud sound with a minimum volume of 85 dB, which makes it ideal for applications where a clearly audible acoustic signal is required. This can be useful, for example, in alarm systems, warning systems or as acoustic feedback in various electronic devices.
Technical Data | |
---|---|
Operating voltage | 3,3 V - 5 V |
Maximum current | 30 mA |
Tone frequency | 2,5kHz ± 300 Hz |
Minimum sound output | 85 dB |
Operating temperature | -20 °C - 70 °C |
Storage temperature | -30 °C - 105 °C |
Dimensions | 19 x 15,5 x 11 mm |
Pin assignment
Micro:Bit | Sensor |
---|---|
Pin 1 | Signal |
- | +V |
GND | GND |
Code-Example
This is an example program which switches the buzzer ON or OFF.
input.onButtonPressed(Button.A, function () {
pins.digitalWritePin(DigitalPin.P1, 1)
basic.pause(500)
pins.digitalWritePin(DigitalPin.P1, 0)
})
Example program download
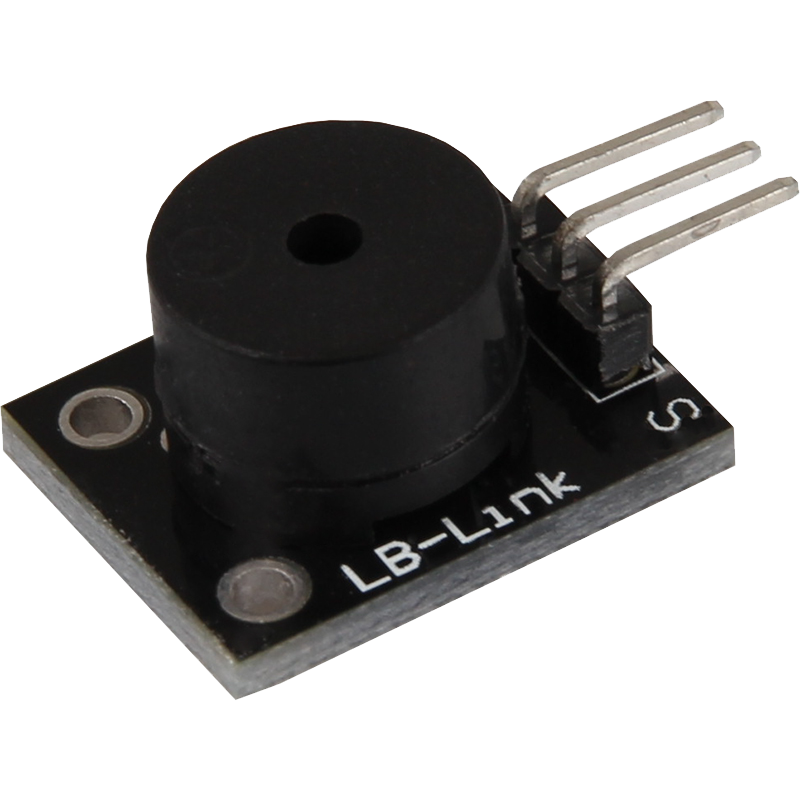
This module contains an active buzzer that immediately generates a sound when it is supplied with voltage. Unlike a passive buzzer, this module does not require an external square-wave voltage to generate a sound. As soon as a voltage of at least 3.3 V is applied to the signal pin, the buzzer automatically generates the necessary square-wave voltage and emits a tone with a frequency of 2.5 kHz.
The buzzer operates in a voltage range of 3.3 V to 5 V and requires a maximum current of 30 mA. It produces a loud sound with a minimum volume of 85 dB, which makes it ideal for applications where a clearly audible acoustic signal is required. This can be useful, for example, in alarm systems, warning systems or as acoustic feedback in various electronic devices.
Technical Data | |
---|---|
Operating voltage | 3,3 V - 5 V |
Maximum current | 30 mA |
Tone frequency | 2,5kHz ± 300 Hz |
Minimum sound output | 85 dB |
Operating temperature | -20 °C - 70 °C |
Storage temperature | -30 °C - 105 °C |
Dimensions | 19 x 15,5 x 11 mm |
Pin assignment
Raspberry Pi Pico | Sensor |
---|---|
GPIO18 | Signal |
- | +V |
GND | GND |
Code example
This code example shows how the buzzer can be alternately switched on for three seconds and then switched off using a definable output pin.
To load the following code example onto your Pico, we recommend using the Thonny IDE. All you have to do first is go to Run > Configure interpreter ... > Interpreter > Which kind of interpreter should Thonny use for running your code? and select MicroPython (Raspberry Pi Pico).
Now copy the code below into your IDE and click on Run.
# Load libraries
from machine import Pin
from utime import sleep
# Initialization of GPIO18 as output
buzzer = Pin(18, Pin.OUT, value=0)
# Switch on buzzer
print("KY-012 Buzzer test")
print("Buzzer on")
buzzer.on()
# wait 3 seconds
sleep(3)
# Switch off buzzer
print("Buzzer off")
buzzer.off()