KY-002 Vibration switch
This sensor is a module that can sense vibrations and emits a signal at each vibration.
- Arduino
- Raspberry Pi
- Raspberry Pi Pico
- Micro:Bit
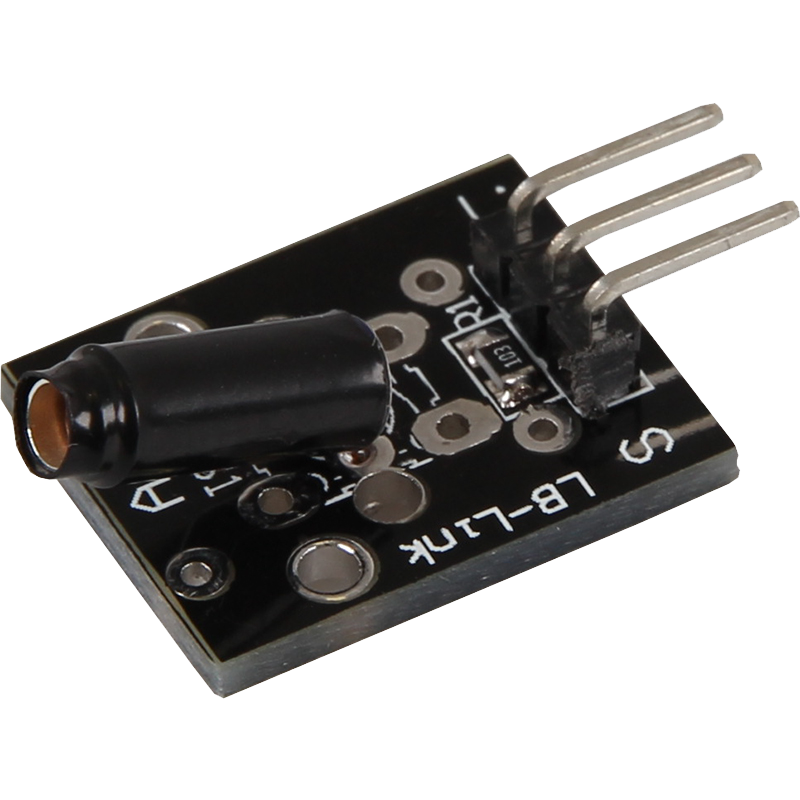
The KY-002 shock and vibration sensor is a precise module for detecting shocks and vibrations. It consists of a conductive outer casing and an internal spring. In the event of shocks, the spring closes the contact to the outer casing and thus generates an electrical signal. This simple design enables reliable and fast detection of vibrations.
Technically speaking, the KY-002 operates in the voltage range from 3.3 V to 5 V. The compact design facilitates integration into a wide variety of projects. Thanks to its robustness and ease of use, the KY-002 is ideal for applications in security systems where vibrations on doors and windows need to be detected, as well as in industrial automation for monitoring machines and systems for unusual vibrations. The sensor can also be used to measure vibrations and shocks in the sports and fitness sector.
Technical Data | |
---|---|
Operating voltage | 3,3 V - 5 V |
Dimensions | 18,5 mm x 15 mm |
Pin assignment
Arduino | Sensor |
---|---|
Pin 10 | Signal |
5 V | +V |
GND | GND |
Code-Example
To load the following code example onto your Arduino, we recommend using the Arduino IDE. In the IDE, you can select the appropriate port and board for your device.
Copy the code below into your IDE. To upload the code to your Arduino, simply click on the upload button.
int vib = 10; // Declaration of the sensor input pin
int value; // Temporary variable
void setup ()
{
pinMode(vib, INPUT); // Initialization sensor pin
digitalWrite(vib, HIGH); // Activation of internal pull-up resistor
Serial.begin(9600); // Initialization of the serial monitor
Serial.print("KY-002 Vibration detection");
}
void loop ()
{
// The current signal at the sensor is read out.
value = digitalRead(vib);
// If a signal could be detected, this is displayed on the serial monitor.
if (value == LOW) {
Serial.println("Signal detected");
delay(100); // 100 ms break
}
}
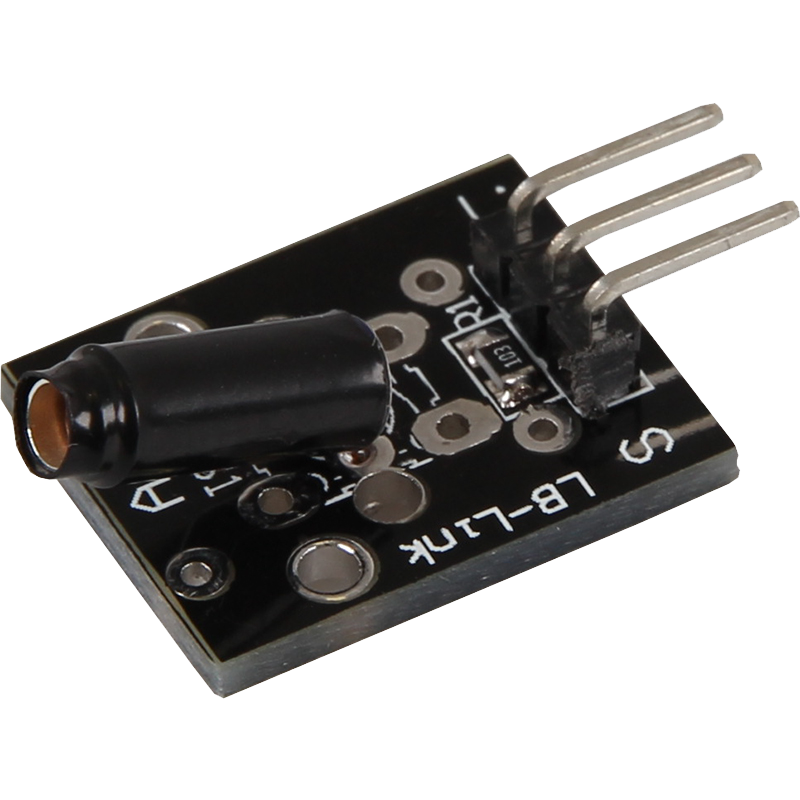
The KY-002 shock and vibration sensor is a precise module for detecting shocks and vibrations. It consists of a conductive outer casing and an internal spring. In the event of shocks, the spring closes the contact to the outer casing and thus generates an electrical signal. This simple design enables reliable and fast detection of vibrations.
Technically speaking, the KY-002 operates in the voltage range from 3.3 V to 5 V. The compact design facilitates integration into a wide variety of projects. Thanks to its robustness and ease of use, the KY-002 is ideal for applications in security systems where vibrations on doors and windows need to be detected, as well as in industrial automation for monitoring machines and systems for unusual vibrations. The sensor can also be used to measure vibrations and shocks in the sports and fitness sector.
Technical Data | |
---|---|
Operating voltage | 3,3 V - 5 V |
Dimensions | 18,5 mm x 15 mm |
Pin assignment
Raspberry Pi | Sensor |
---|---|
GPIO 24 [Pin 18] | Signal |
3.3 V [Pin 1] | +V |
GND [Pin 6] | GND |
Code-Example
This is an example program that prints a console output when as signal is detected at the sensor.
from gpiozero import Button
import time
# The input pin to which the sensor is connected is declared here.
# The button object uses the internal pull-up resistor of the Pi.
sensor = Button(24, pull_up=True)
print("Sensor test [press CTRL+C to end the test]")
# This output function is executed when a signal is detected
def ausgabeFunktion():
print("Signal recognized")
# When a signal is detected (falling signal edge), the output function is triggered
sensor.when_pressed = ausgabeFunktion
# Main program loop
try:
while True:
time.sleep(1)
# Clean-up work after the program has been completed
except KeyboardInterrupt:
print("Program terminated")
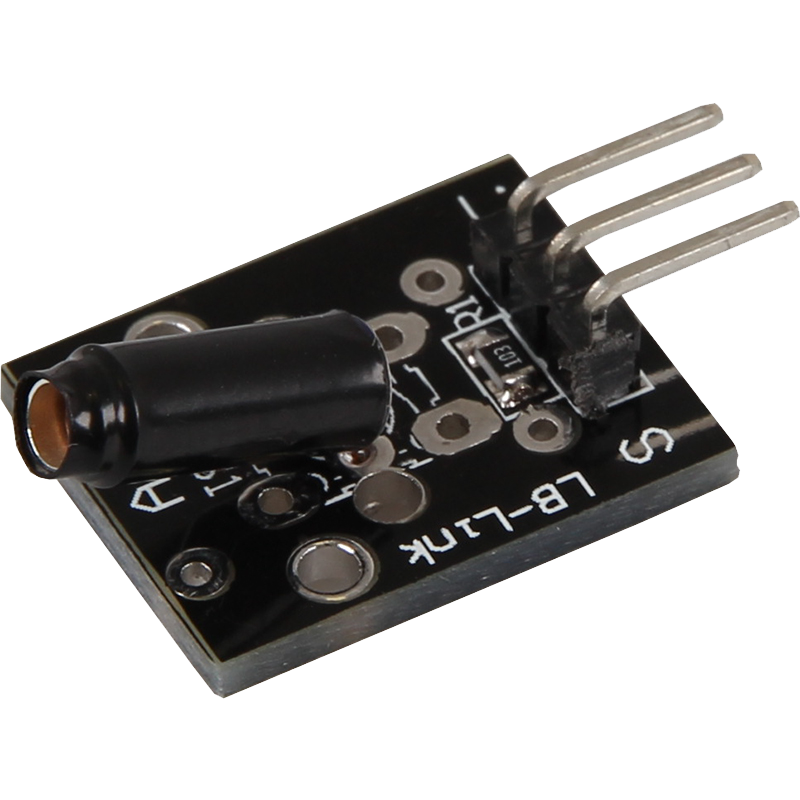
The KY-002 shock and vibration sensor is a precise module for detecting shocks and vibrations. It consists of a conductive outer casing and an internal spring. In the event of shocks, the spring closes the contact to the outer casing and thus generates an electrical signal. This simple design enables reliable and fast detection of vibrations.
Technically speaking, the KY-002 operates in the voltage range from 3.3 V to 5 V. The compact design facilitates integration into a wide variety of projects. Thanks to its robustness and ease of use, the KY-002 is ideal for applications in security systems where vibrations on doors and windows need to be detected, as well as in industrial automation for monitoring machines and systems for unusual vibrations. The sensor can also be used to measure vibrations and shocks in the sports and fitness sector.
Technical Data | |
---|---|
Operating voltage | 3,3 V - 5 V |
Dimensions | 18,5 mm x 15 mm |
Pin assignment
Micro:Bit | Sensor |
---|---|
Pin 1 | Signal |
3 V | +V |
GND | GND |
Code-Example
pins.setPull(DigitalPin.P1, PinPullMode.PullDown)
basic.forever(function () {
serial.writeLine("" + (pins.digitalReadPin(DigitalPin.P1)))
if (pins.digitalReadPin(DigitalPin.P1) == 1) {
serial.writeLine("Shock detected")
} else {
serial.writeLine("No shock detected")
}
serial.writeLine("_____________________________________")
basic.pause(1000)
})
Example program download
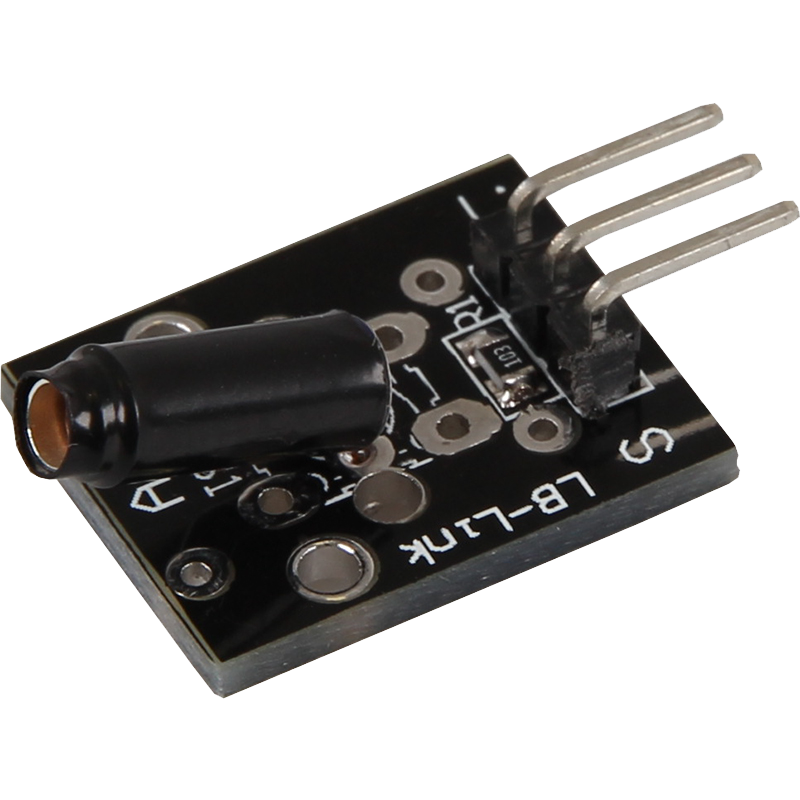
The KY-002 shock and vibration sensor is a precise module for detecting shocks and vibrations. It consists of a conductive outer casing and an internal spring. In the event of shocks, the spring closes the contact to the outer casing and thus generates an electrical signal. This simple design enables reliable and fast detection of vibrations.
Technically speaking, the KY-002 operates in the voltage range from 3.3 V to 5 V. The compact design facilitates integration into a wide variety of projects. Thanks to its robustness and ease of use, the KY-002 is ideal for applications in security systems where vibrations on doors and windows need to be detected, as well as in industrial automation for monitoring machines and systems for unusual vibrations. The sensor can also be used to measure vibrations and shocks in the sports and fitness sector.
Technical Data | |
---|---|
Operating voltage | 3,3 V - 5 V |
Dimensions | 18,5 mm x 15 mm |
Pin assignment
Raspberry Pi Pico | Sensor |
---|---|
GPIO26 | Signal |
3.3V | +V |
GND | GND |
Code-Example
This is an example program that counts up and outputs text serially when a signal is detected at the sensor.
To load the following code example onto your Pico, we recommend using the Thonny IDE. All you have to do first is go to Run > Configure interpreter ... > Interpreter > Which kind of interpreter should Thonny use for running your code? and select MicroPython (Raspberry Pi Pico).
Now copy the code below into your IDE and click on Run.
# Load libraries
from machine import Pin, Timer
# Initialization of GPIO26 as input
sensor = Pin(26, Pin.IN, Pin.PULL_DOWN)
# Create timer
timer = Timer()
# Initialization of the counter variable
counter = 0
print("KY-002 Vibration sensor")
# Function for counting the vibrations
def step(timer):
global counter
counter = counter + 1
print(counter)
# Function that is executed on vibration
def shake(pin):
# Set debounce function around timer
timer.init(mode=Timer.ONE_SHOT, period=300, callback=step)
# Initialization of the interrupt
sensor.irq(trigger=Pin.IRQ_FALLING, handler=shake)