KY-050 Ultrasonic distance sensor
This sensor is used for distance measurement via ultrasound.
- Arduino
- Raspberry Pi
- Raspberry Pi Pico
- Micro:Bit
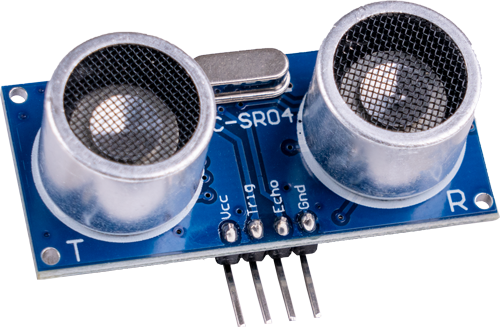
This module is ideal for distance measurement in a range between 2 cm and 3 m. With a resolution of around 3 mm, distances can be measured precisely using an ultrasonic signal. When a signal (falling edge) is applied to the trigger input, the sensor starts a distance measurement. The result is output at the echo output as a PWM TTL signal.
The ultrasonic distance sensor is particularly useful for obstacle detection, distance measurement, level indicators and various industrial applications. Thanks to its high precision and fast response time (minimum time between measurements is 50 µs), the sensor can operate reliably in real time.
This module is versatile and offers a simple and effective solution for projects where accurate distance measurements are required.
Technical Specifications | |
---|---|
Measurable distance | 2 cm - 300 cm |
Measurement resolution | 3 mm |
Min. time between measurements | 50 µs |
Principle of operation
This module shows how an ultrasonic loudspeaker and a microphone can be used to measure the distance to an object without contact. The principle is based on the fact that the speed of sound in air remains almost constant at constant temperature - at 20 °C it is 343.2 m / s.
From this, the distance measurement can be converted into a time measurement, which can then easily be taken over by microcontrollers.
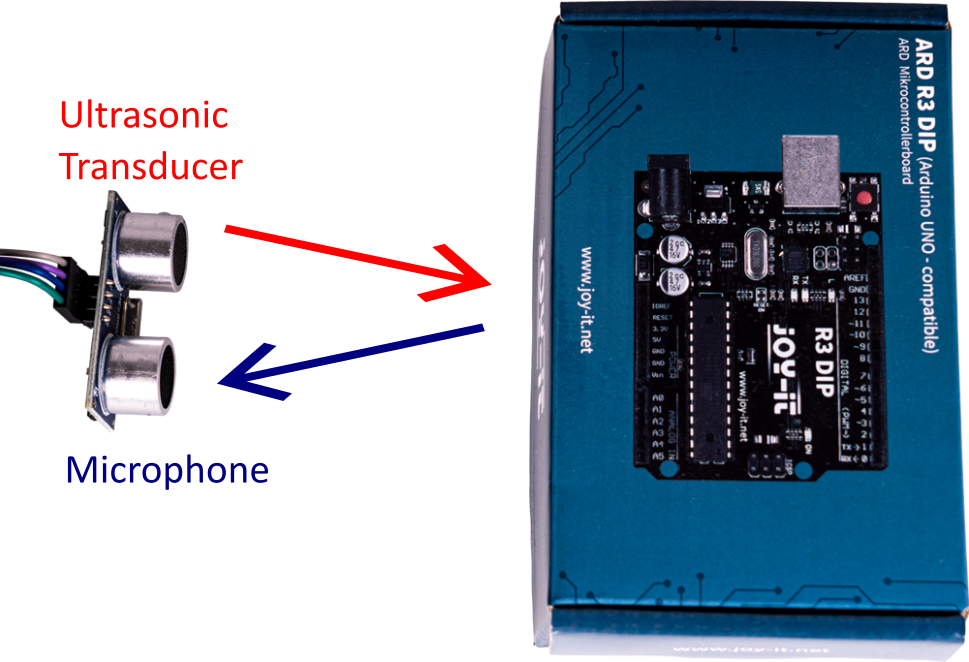
When triggered, the ultrasonic loudspeaker (transducer) emits an ultrasonic noise of maximum 200µs. The ultrasonic loudspeaker emits a 40 kHz signal. This means that 8 periods (edge changes) are emitted within the 200µs in which the sensor emits its ultrasonic noise. In order to arrive at these 8 periods of the 40 kHz signal mathematically, one calculates the whole thing as shown in the following.
Number of periods in one second = 40000
Time = 1s
Duration of a single period:
1s / 40000 = 25µs**
Maximum length of ultrasonic sound = 200us
Duration of a single period = 25us
Number of periods in ultrasonic sound:
200µs / 25µs = 8
The principle is kept simple, an ultrasonic sound is emitted from the speaker on the PCB, which is then reflected from an object and picked up by the microphone on the PCB. Ultrasound is used because it is outside the hearing range of the human ear (about 20Hz-22kHz).
Transmission of the ultrasonic signal is started when a 10µs long start signal (ActiveHigh) is received at the "Trigger input pin". After transmission, the signal is activated at the "Echo output signal pin" (ActiveHigh). If the reflected signal is now picked up again at the microphone, the echo signal is deactivated again after detection. The time between activation and deactivation of the echo signal can be measured and converted to distance, as this also corresponds to how long it takes the ultrasonic signal to cover the distance between loudspeaker-> reflecting wall -> microphone in the air. The conversion is then made by approximating a constant air velocity - the distance is then half the distance traveled.
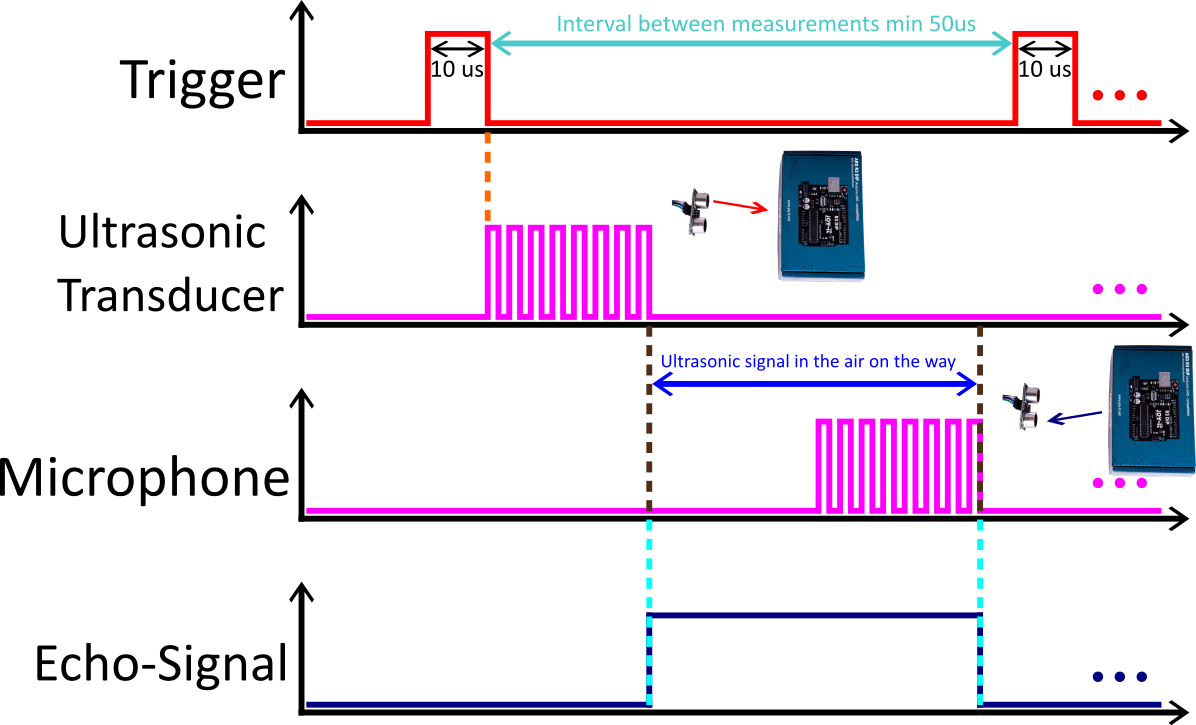
Pin assignment
Arduino | Sensor |
---|---|
Pin 7 | Echo |
5 V | + V |
GND | GND |
Pin 8 | Trigger |
Code example
The example program activates the distance measurement according to the above principle and measures the time until the ultrasonic signal is picked up by the microphone with the help of the Arduino function pulseIn . This time is then taken as the basis for the conversion of the distance - the result is then output in the serial output. If the signal is outside the measuring range, a corresponding error message is output.
To load the following code example onto your Arduino, we recommend using the Arduino IDE. In the IDE, you can select the appropriate port and board for your device.
Copy the code below into your IDE. To upload the code to your Arduino, simply click on the upload button.
#define echoPin 7 // Echo input pin
#define triggerPin 8 // Trigger output pin
// Required variables are defined
int maximumRange = 300;
int minimumRange = 2;
long distance;
long duration;
void setup() {
pinMode(triggerPin, OUTPUT);
pinMode(echoPin, INPUT);
Serial.begin(9600);
Serial.println("KY-050 Distance measurement");
}
void loop() {
// Distance measurement is started using the 10us trigger signal
digitalWrite(triggerPin, HIGH);
delayMicroseconds(10);
digitalWrite(triggerPin, LOW);
// Now wait at the echo input until the signal has been activated
// and then the time is measured to see how long it remains activated
duration = pulseIn(echoPin, HIGH);
// Now the distance is calculated using the recorded time
distance = duration/58.2;
// Check whether the measured value is within the permissible distance
if (distance >= maximumRange || distance <= minimumRange) {
// If not, an error message is displayed.
Serial.println("Distance outside the measuring range");
Serial.println("-----------------------------------");
}
else {
// The calculated distance is output in the serial output
Serial.print("The distance is: ");
Serial.print(distance);
Serial.println(" cm");
Serial.println("-----------------------------------");
}
// Pause between the individual measurements
delay(500);
}
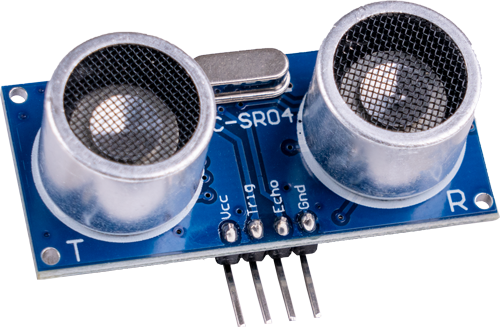
This module is ideal for distance measurement in a range between 2 cm and 3 m. With a resolution of around 3 mm, distances can be measured precisely using an ultrasonic signal. When a signal (falling edge) is applied to the trigger input, the sensor starts a distance measurement. The result is output at the echo output as a PWM TTL signal.
The ultrasonic distance sensor is particularly useful for obstacle detection, distance measurement, level indicators and various industrial applications. Thanks to its high precision and fast response time (minimum time between measurements is 50 µs), the sensor can operate reliably in real time.
This module is versatile and offers a simple and effective solution for projects where accurate distance measurements are required.
Technical Specifications | |
---|---|
Measurable distance | 2 cm - 300 cm |
Measurement resolution | 3 mm |
Min. time between measurements | 50 µs |
Principle of operation
This module shows how an ultrasonic loudspeaker and a microphone can be used to measure the distance to an object without contact. The principle is based on the fact that the speed of sound in air remains almost constant at constant temperature - at 20 °C it is 343.2 m / s.
From this, the distance measurement can be converted into a time measurement, which can then easily be taken over by microcontrollers.
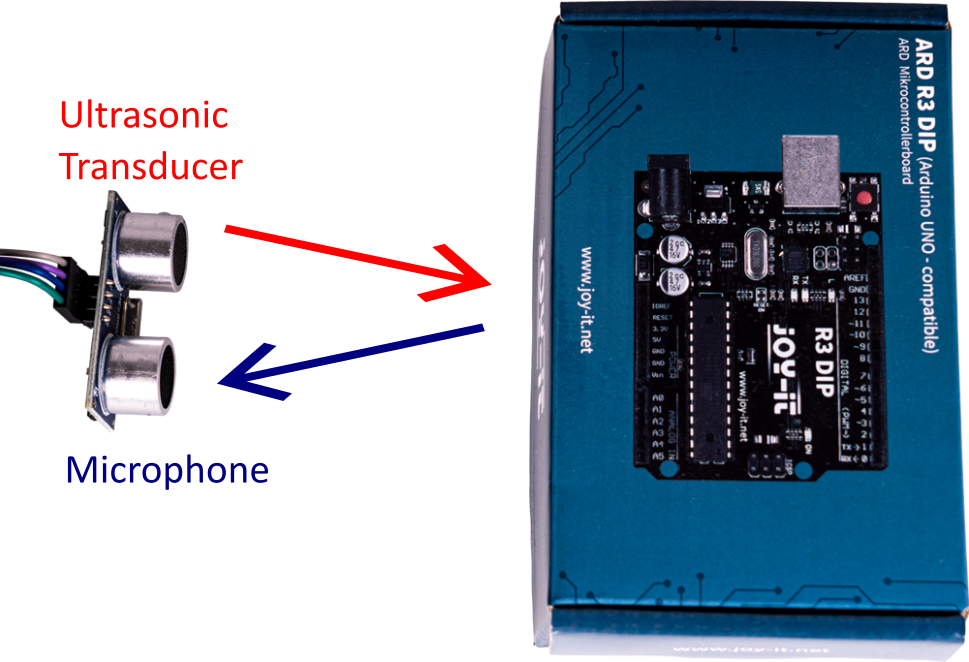
When triggered, the ultrasonic loudspeaker (transducer) emits an ultrasonic noise of maximum 200µs. The ultrasonic loudspeaker emits a 40 kHz signal. This means that 8 periods (edge changes) are emitted within the 200µs in which the sensor emits its ultrasonic noise. In order to arrive at these 8 periods of the 40 kHz signal mathematically, one calculates the whole thing as shown in the following.
Number of periods in one second = 40000
Time = 1s
Duration of a single period:
1s / 40000 = 25µs**
Maximum length of ultrasonic sound = 200us
Duration of a single period = 25us
Number of periods in ultrasonic sound:
200µs / 25µs = 8
The principle is kept simple, an ultrasonic sound is emitted from the speaker on the PCB, which is then reflected from an object and picked up by the microphone on the PCB. Ultrasound is used because it is outside the hearing range of the human ear (about 20Hz-22kHz).
Transmission of the ultrasonic signal is started when a 10µs long start signal (ActiveHigh) is received at the "Trigger input pin". After transmission, the signal is activated at the "Echo output signal pin" (ActiveHigh). If the reflected signal is now picked up again at the microphone, the echo signal is deactivated again after detection. The time between activation and deactivation of the echo signal can be measured and converted to distance, as this also corresponds to how long it takes the ultrasonic signal to cover the distance between loudspeaker-> reflecting wall -> microphone in the air. The conversion is then made by approximating a constant air velocity - the distance is then half the distance traveled.
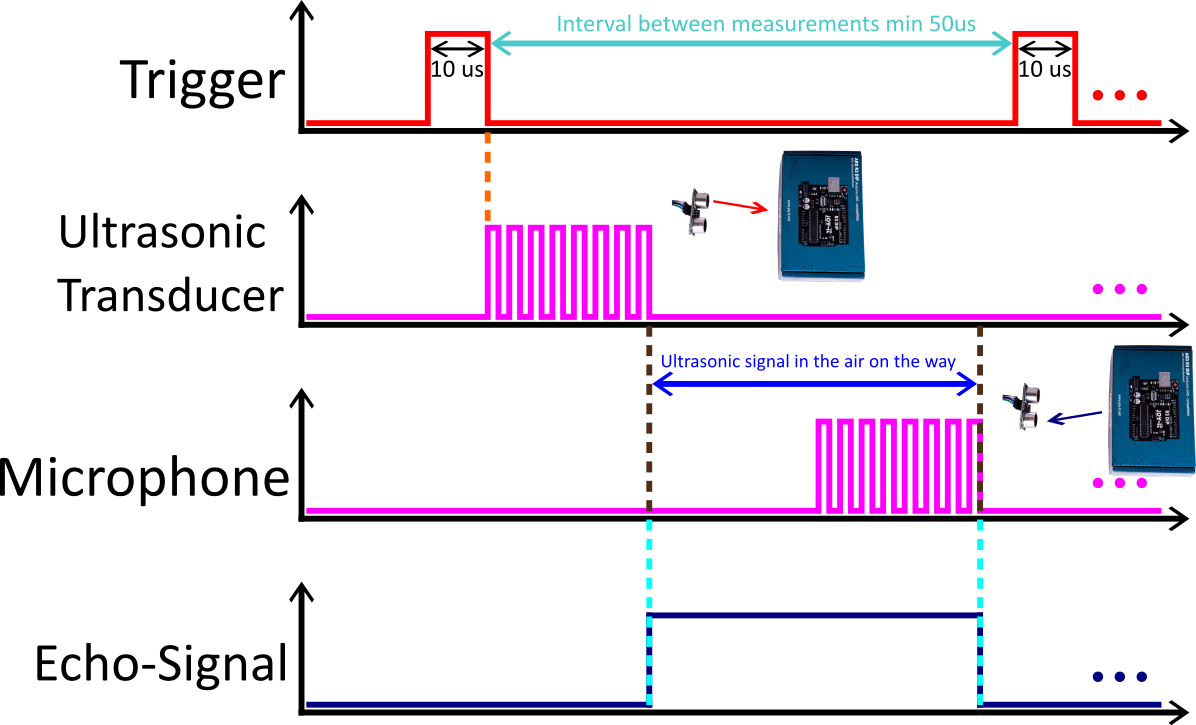
Pin assignment
Raspberry Pi | Sensor |
---|---|
5 V [Pin 2] | +V |
Masse [Pin 6] | GND |
- | Trigger |
- | Echo |
Sensor | KY-051 |
---|---|
Trigger | B1 |
Echo | B2 |
+V | - |
GND | - |
Raspberry Pi | KY-051 |
---|---|
3,3 V [Pin 1] | Vcca |
5 V [Pin 4] | Vccb |
GND [Pin 14] | GND |
GPIO 17 [Pin 11] | A1 |
GPIO 27 [Pin 13] | A2 |
Code example
5V voltage level, therefore the following has to be considered: The Raspberry Pi works with its ARM processor core, unlike the Atmel Atmega based Arduino, with 3.3V voltage level instead of 5V. However, this sensor only works with the higher voltage level. If the sensor would be used without any restrictions on the Raspberry Pi, without any precautions, this could cause permanent damage to the Raspberry Pi.
To prevent this, it is recommended to connect a so-called voltage translator in between, which adjusts the voltage levels accordingly and ensures a safe operation. Our KY-051 Voltage Translator is perfectly suited for this purpose.
The example program activates the distance measurement according to the above principle and measures the time how long the ultrasonic signal is in the air. This time is then taken as a basis for the conversion of the distance - the result is then output in the cosnole. If the signal is outside the measuring range, a corresponding error message is output.
from gpiozero import DistanceSensor
import time
# Define the pins for the sensor
trigger_pin = 17
echo_pin = 27
# The pause between the individual measurements can be set here in seconds
sleeptime = 0.8
# Initialize the ultrasonic sensor
sensor = DistanceSensor(echo=echo_pin, trigger=trigger_pin)
# Main program loop
try:
while True:
# Measures the distance and outputs it
distance = sensor.distance * 100 # Conversion from meters to centimeters
if distance < 2 or distance > 300:
print("Distance outside the measuring range")
print("------------------------------")
else:
# The spacing is formatted to two decimal places
print(f"The distance is: {distance:.2f} cm")
print("------------------------------")
# Pause between the individual measurements
time.sleep(sleeptime)
# Clean up after the program has been completed
except KeyboardInterrupt:
print("Program was interrupted by user”)
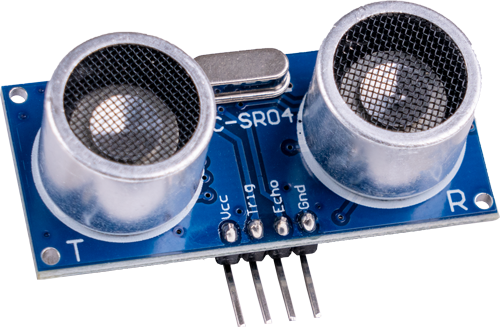
This module is ideal for distance measurement in a range between 2 cm and 3 m. With a resolution of around 3 mm, distances can be measured precisely using an ultrasonic signal. When a signal (falling edge) is applied to the trigger input, the sensor starts a distance measurement. The result is output at the echo output as a PWM TTL signal.
The ultrasonic distance sensor is particularly useful for obstacle detection, distance measurement, level indicators and various industrial applications. Thanks to its high precision and fast response time (minimum time between measurements is 50 µs), the sensor can operate reliably in real time.
This module is versatile and offers a simple and effective solution for projects where accurate distance measurements are required.
Technical Specifications | |
---|---|
Measurable distance | 2 cm - 300 cm |
Measurement resolution | 3 mm |
Min. time between measurements | 50 µs |
Principle of operation
This module shows how an ultrasonic loudspeaker and a microphone can be used to measure the distance to an object without contact. The principle is based on the fact that the speed of sound in air remains almost constant at constant temperature - at 20 °C it is 343.2 m / s.
From this, the distance measurement can be converted into a time measurement, which can then easily be taken over by microcontrollers.
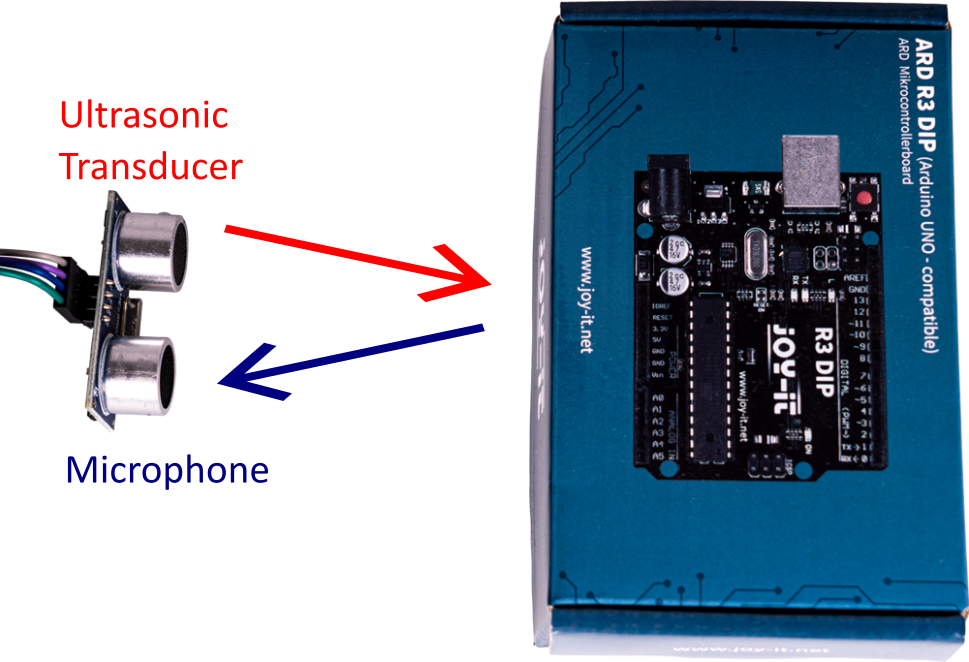
When triggered, the ultrasonic loudspeaker (transducer) emits an ultrasonic noise of maximum 200µs. The ultrasonic loudspeaker emits a 40 kHz signal. This means that 8 periods (edge changes) are emitted within the 200µs in which the sensor emits its ultrasonic noise. In order to arrive at these 8 periods of the 40 kHz signal mathematically, one calculates the whole thing as shown in the following.
Number of periods in one second = 40000
Time = 1s
Duration of a single period:
1s / 40000 = 25µs**
Maximum length of ultrasonic sound = 200us
Duration of a single period = 25us
Number of periods in ultrasonic sound:
200µs / 25µs = 8
The principle is kept simple, an ultrasonic sound is emitted from the speaker on the PCB, which is then reflected from an object and picked up by the microphone on the PCB. Ultrasound is used because it is outside the hearing range of the human ear (about 20Hz-22kHz).
Transmission of the ultrasonic signal is started when a 10µs long start signal (ActiveHigh) is received at the "Trigger input pin". After transmission, the signal is activated at the "Echo output signal pin" (ActiveHigh). If the reflected signal is now picked up again at the microphone, the echo signal is deactivated again after detection. The time between activation and deactivation of the echo signal can be measured and converted to distance, as this also corresponds to how long it takes the ultrasonic signal to cover the distance between loudspeaker-> reflecting wall -> microphone in the air. The conversion is then made by approximating a constant air velocity - the distance is then half the distance traveled.
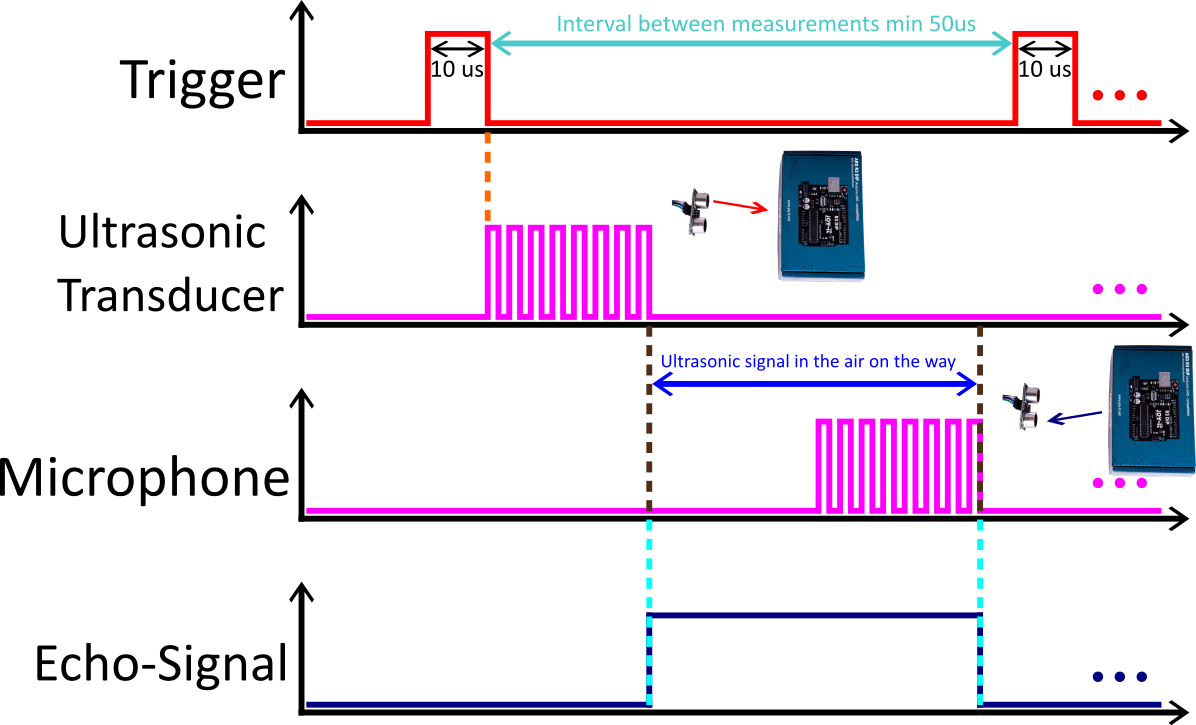
Pin assignment
External | Sensor |
---|---|
External 5V | +V |
Micro:Bit GND + External GND | GND |
- | Trigger |
- | Echo |
Sensor | KY-051 |
---|---|
Trigger | B1 |
Echo | B2 |
+V | - |
GND | - |
Micro:Bit | KY-051 |
---|---|
3,3 V | Vcca |
- | Vccb |
GND + External GND | GND |
Pin 2 | A1 |
Pin 1 | A2 |
External | KY-051 |
---|---|
External 5V | Vccb |
Code example
For the following code example an additional library is needed:
pxt-sonar by Microsoft | published under the MIT License
Add the library to your IDE by clicking on "Extensions" and entering the following URL in the search box: https: //github.com/microsoft/pxt-sonar.git. Confirm the search with [Enter].
let p = 0
basic.forever(function () {
p = sonar.ping(
DigitalPin.P1,
DigitalPin.P2,
PingUnit.Centimeters
)
led.plotBarGraph(
p,
0
)
})
Sample program download
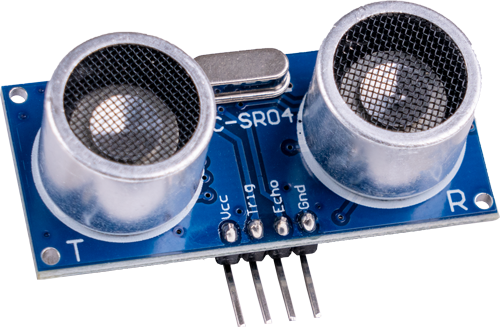
This module is ideal for distance measurement in a range between 2 cm and 3 m. With a resolution of around 3 mm, distances can be measured precisely using an ultrasonic signal. When a signal (falling edge) is applied to the trigger input, the sensor starts a distance measurement. The result is output at the echo output as a PWM TTL signal.
The ultrasonic distance sensor is particularly useful for obstacle detection, distance measurement, level indicators and various industrial applications. Thanks to its high precision and fast response time (minimum time between measurements is 50 µs), the sensor can operate reliably in real time.
This module is versatile and offers a simple and effective solution for projects where accurate distance measurements are required.
Technical Specifications | |
---|---|
Measurable distance | 2 cm - 300 cm |
Measurement resolution | 3 mm |
Min. time between measurements | 50 µs |
Principle of operation
This module shows how an ultrasonic loudspeaker and a microphone can be used to measure the distance to an object without contact. The principle is based on the fact that the speed of sound in air remains almost constant at constant temperature - at 20 °C it is 343.2 m / s.
From this, the distance measurement can be converted into a time measurement, which can then easily be taken over by microcontrollers.
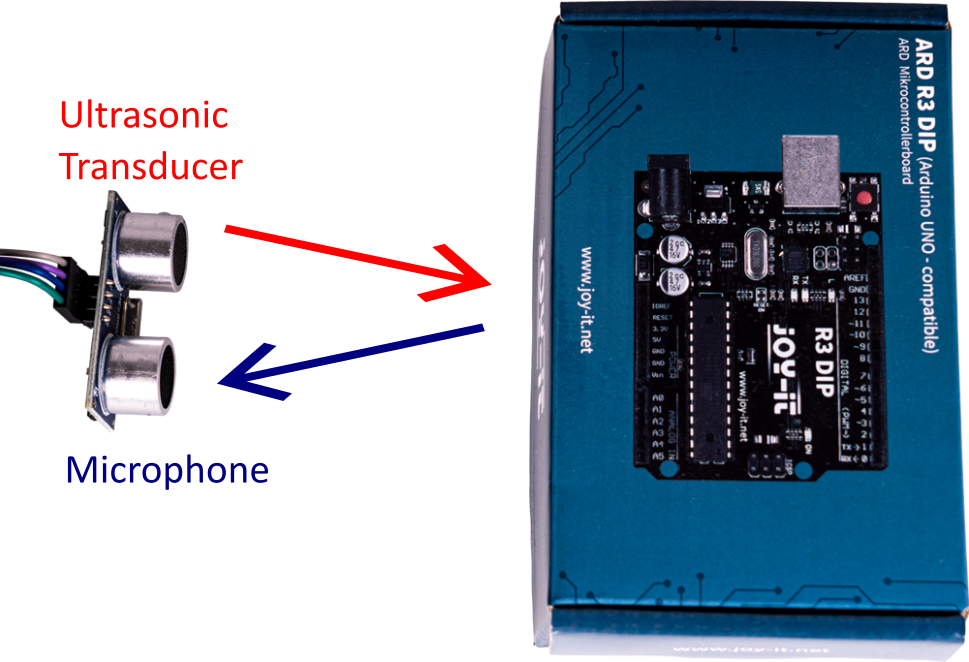
When triggered, the ultrasonic loudspeaker (transducer) emits an ultrasonic noise of maximum 200µs. The ultrasonic loudspeaker emits a 40 kHz signal. This means that 8 periods (edge changes) are emitted within the 200µs in which the sensor emits its ultrasonic noise. In order to arrive at these 8 periods of the 40 kHz signal mathematically, one calculates the whole thing as shown in the following.
Number of periods in one second = 40000
Time = 1s
Duration of a single period:
1s / 40000 = 25µs**
Maximum length of ultrasonic sound = 200us
Duration of a single period = 25us
Number of periods in ultrasonic sound:
200µs / 25µs = 8
The principle is kept simple, an ultrasonic sound is emitted from the speaker on the PCB, which is then reflected from an object and picked up by the microphone on the PCB. Ultrasound is used because it is outside the hearing range of the human ear (about 20Hz-22kHz).
Transmission of the ultrasonic signal is started when a 10µs long start signal (ActiveHigh) is received at the "Trigger input pin". After transmission, the signal is activated at the "Echo output signal pin" (ActiveHigh). If the reflected signal is now picked up again at the microphone, the echo signal is deactivated again after detection. The time between activation and deactivation of the echo signal can be measured and converted to distance, as this also corresponds to how long it takes the ultrasonic signal to cover the distance between loudspeaker-> reflecting wall -> microphone in the air. The conversion is then made by approximating a constant air velocity - the distance is then half the distance traveled.
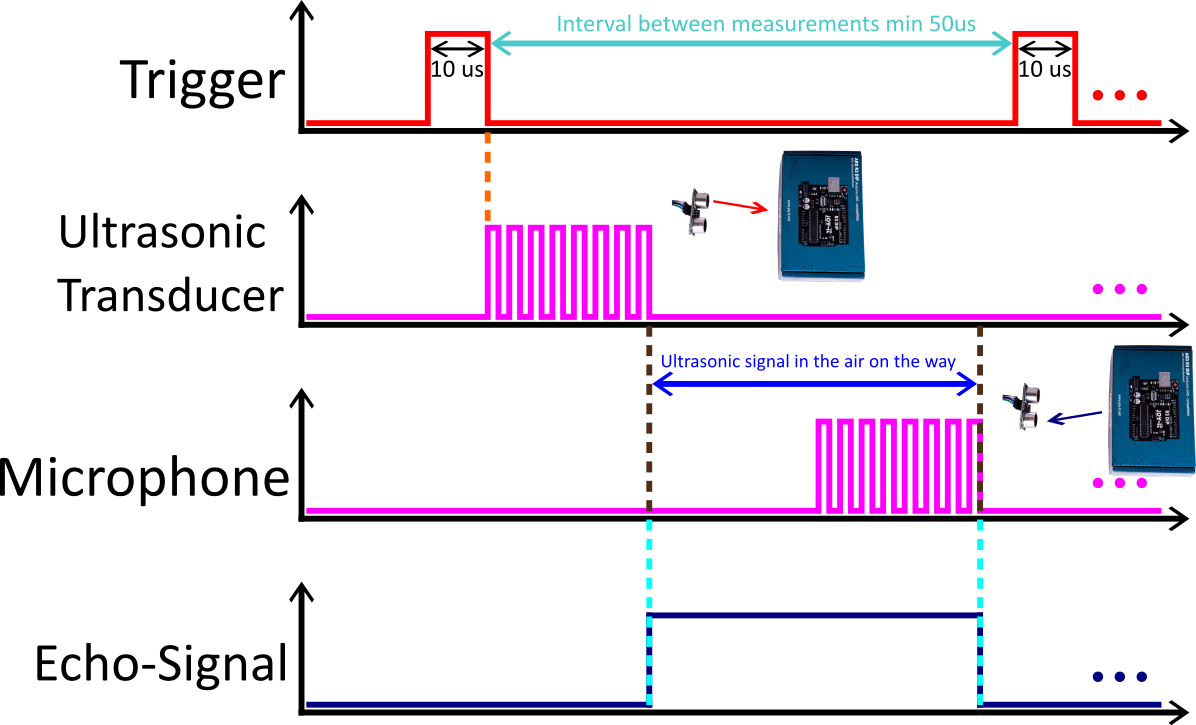
Pin assignment
Since the KY-050 is operated with 5V, a voltage translator and an external power supply are required to operate it with the Raspberry Pi Pico. You can use KY-051 as a voltage translator and an Arduino or Raspberry Pi, for example, as an external power supply.
Voltage Translator | KY-050 |
---|---|
Vccb | +V |
GND | GND |
B1 | Echo |
B2 | Trig |
Raspberry Pi Pico | External |
---|---|
GND | External GND |
Voltage Translator | External |
---|---|
Vccb | External 5V |
GND | External GND |
Voltage Translator | Raspberry Pi Pico |
---|---|
Vcca | 3,3V |
GND | GND |
A1 | GPIO16 |
A2 | GPIO17 |
Code example
The example program activates the distance measurement according to the above principle and calculates the time until the ultrasonic signal is picked up by the microphone. This time is then taken as a basis for the conversion of the distance - the result is then output in the serial output. If the signal is outside the measuring range, a corresponding error message is output.
To load the following code example onto your Pico, we recommend using the Thonny IDE. All you have to do first is go to Run > Configure interpreter ... > Interpreter > Which kind of interpreter should Thonny use for running your code? and select MicroPython (Raspberry Pi Pico).
Now copy the code below into your IDE and click on Run.
# Load libraries
from machine import Pin
import time
# Initialization of GPIO16 as input and GPIO17 as output
trigger_pin = Pin(17, Pin.OUT)
echo_pin = Pin(16, Pin.IN, Pin.PULL_DOWN)
print("KY-050 Distance measurement")
# Endless loop for measuring the distance
while True:
# Distance measurement is started using the 10us trigger signal
trigger_pin.value(0)
time.sleep(0.1)
trigger_pin.value(1)
# Now wait at the echo input until the signal has been activated
# Then the time is measured for how long it remains activated
time.sleep_us(2)
trigger_pin.value(0)
while echo_pin.value()==0:
pulse_start = time.ticks_us()
while echo_pin.value()==1:
pulse_end = time.ticks_us()
pulse_duration = pulse_end - pulse_start
# Now the distance is calculated using the recorded time
distance = pulse_duration * 17165 / 1000000
distance = round(distance, 0)
# Serial output
print ('Distance:',"{:.0f}".format(distance),'cm')
time.sleep(1)