KY-027 Magic light cup
The LED is turned on or off when there is a vibration.
- Arduino
- Raspberry Pi
- Raspberry Pi Pico
- Micro:Bit
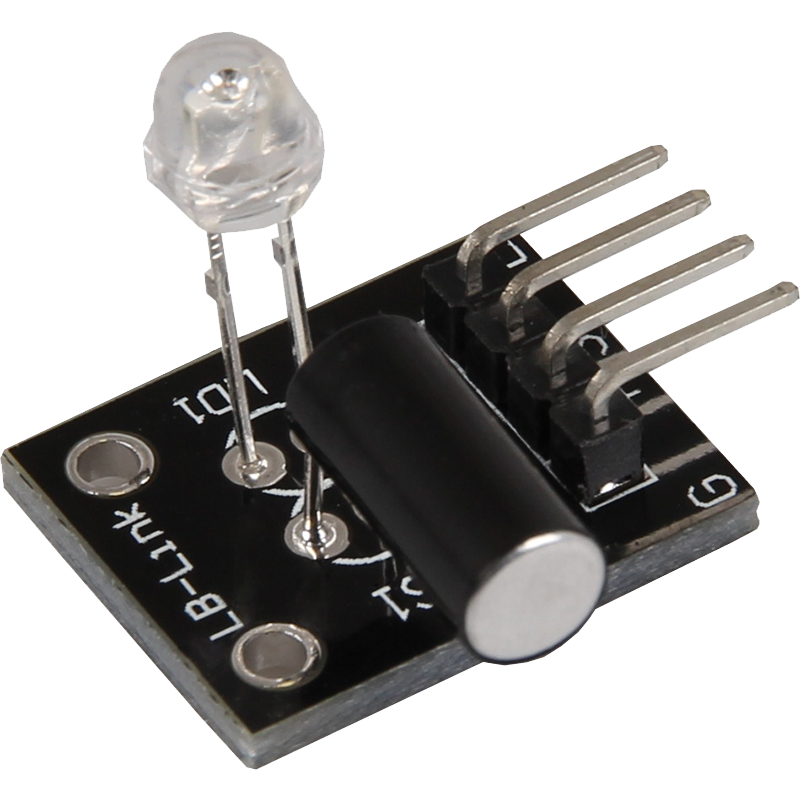
The Magic Light Cup module switches the LED on or off when a shock is detected. If a vibration is detected, the LED is activated or deactivated and the corresponding control signal is forwarded to a signal output. This signal can be read out to monitor the status of the LED. The module is ideal for applications where the reaction to movement or vibration is used, such as interactive lights, toys or alarm systems. By forwarding the trigger signal to the signal output, other systems or microcontrollers can detect the vibrations and react accordingly.
Series resistor:
Depending on the input voltage, series resistors are required.
Input voltage | Series resistor |
---|---|
3.3 V | 120 Ω |
5 V | 220 Ω |
Pin assignment
Arduino | Sensor |
---|---|
Pin 10 | signal |
5 V | +V |
GND | GND |
Pin 13 | LED |
Code example
To load the following code example onto your Arduino, we recommend using the Arduino IDE. In the IDE, you can select the appropriate port and board for your device.
Copy the code below into your IDE. To upload the code to your Arduino, simply click on the upload button.
int led = 13 ;// Declaration of the LED output pin
int shock_sensor = 10; // Declaration of the sensor input pin
int value; // Temporary variable
void setup () {
pinMode(led, OUTPUT); // Initialization output pin
pinMode(shock_sensor, INPUT); // Initialization sensor pin
digitalWrite(shock_sensor, HIGH); // Activation of internal pull-up resistor
}
void loop () {
value = digitalRead(shock_sensor); // The current signal at the sensor is read out
// If a signal is detected, the LED is switched on.
if (value == HIGH) {
digitalWrite (led, LOW);
delay(200);
}
else digitalWrite(led, HIGH);
}
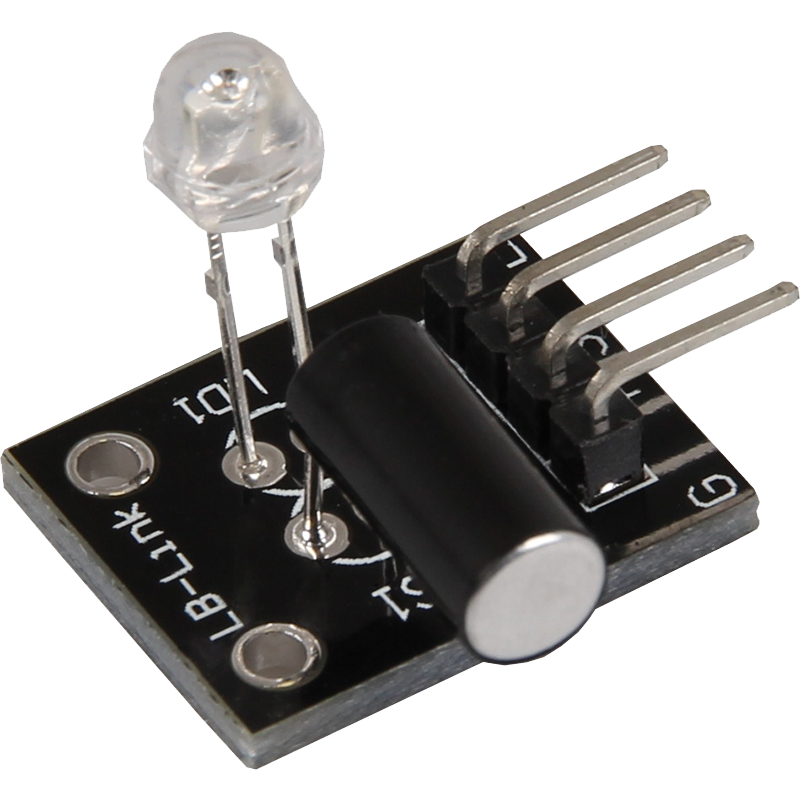
The Magic Light Cup module switches the LED on or off when a shock is detected. If a vibration is detected, the LED is activated or deactivated and the corresponding control signal is forwarded to a signal output. This signal can be read out to monitor the status of the LED. The module is ideal for applications where the reaction to movement or vibration is used, such as interactive lights, toys or alarm systems. By forwarding the trigger signal to the signal output, other systems or microcontrollers can detect the vibrations and react accordingly.
Series resistor:
Depending on the input voltage, series resistors are required.
Input voltage | Series resistor |
---|---|
3.3 V | 120 Ω |
5 V | 220 Ω |
Pin assignment
Raspberry Pi | Sensor |
---|---|
GPIO 23 [Pin 16] | Signal |
5 V | +V |
GND | GND |
GPIO 24 [Pin 18] | LED |
Code example
# Required modules are imported and set up
from gpiozero import LED
import time
# Initialization of the LEDs
led_red = LED(24)
led_green = LED(23)
print("LED test [press CTRL+C to end the test]")
# Main program loop
try:
while True:
print("LED RED on for 3 seconds")
led_red.on() # Red LED is switched on
led_green.off() # Green LED is switched off
time.sleep(3) # Wait mode for 3 seconds
print("LED GREEN on for 3 seconds")
led_red.off() # Red LED is switched off
led_green.on() # Green LED is switched on
time.sleep(3) # Wait mode for 3 seconds
# Clean-up work after the program has been completed
except KeyboardInterrupt:
print("Program was interrupted by user")
led_red.close() # Release resources for red LED
led_green.close() # Release resources for green LED
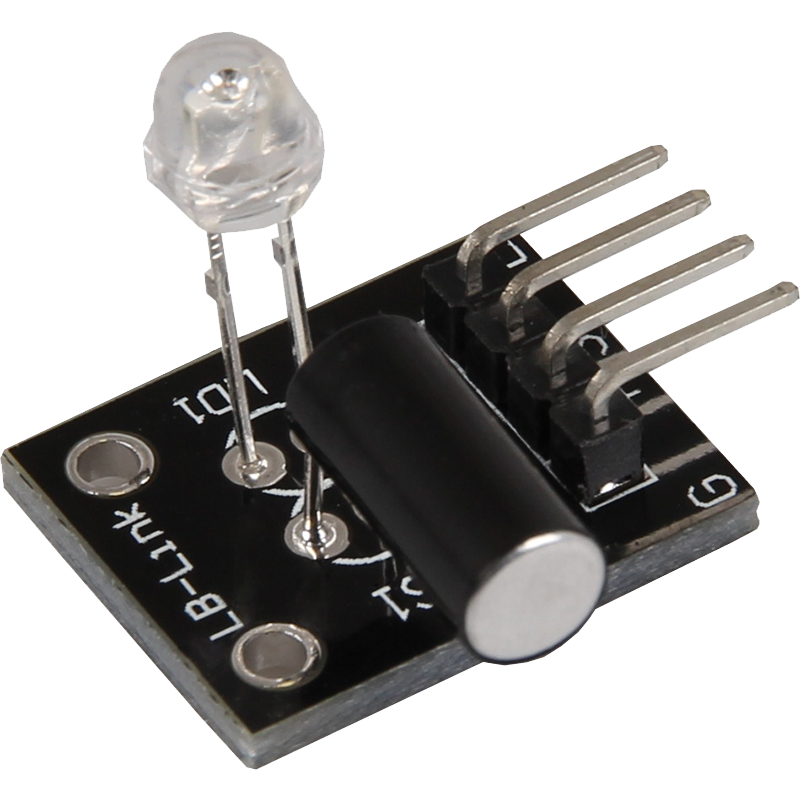
The Magic Light Cup module switches the LED on or off when a shock is detected. If a vibration is detected, the LED is activated or deactivated and the corresponding control signal is forwarded to a signal output. This signal can be read out to monitor the status of the LED. The module is ideal for applications where the reaction to movement or vibration is used, such as interactive lights, toys or alarm systems. By forwarding the trigger signal to the signal output, other systems or microcontrollers can detect the vibrations and react accordingly.
Series resistor:
Depending on the input voltage, series resistors are required.
Input voltage | Series resistor |
---|---|
3.3 V | 120 Ω |
5 V | 220 Ω |
Pin assignment
Micro:Bit | Sensor |
---|---|
Pin 2 | LED |
Pin 1 | Signal |
3 V | +V |
GND | GND |
Code example
basic.forever(function () {
if (pins.digitalReadPin(DigitalPin.P1) == 1) {
pins.digitalWritePin(DigitalPin.P2, 1)
} else {
pins.digitalWritePin(DigitalPin.P2, 0)
}
})
Sample program download
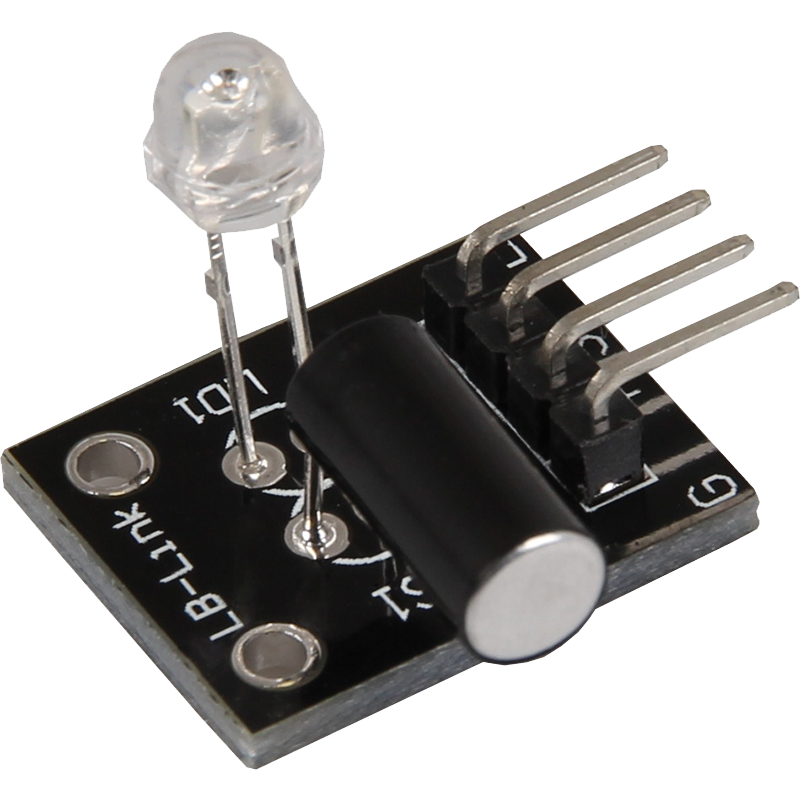
The Magic Light Cup module switches the LED on or off when a shock is detected. If a vibration is detected, the LED is activated or deactivated and the corresponding control signal is forwarded to a signal output. This signal can be read out to monitor the status of the LED. The module is ideal for applications where the reaction to movement or vibration is used, such as interactive lights, toys or alarm systems. By forwarding the trigger signal to the signal output, other systems or microcontrollers can detect the vibrations and react accordingly.
Series resistor:
Depending on the input voltage, series resistors are required.
Input voltage | Series resistor |
---|---|
3.3 V | 120 Ω |
5 V | 220 Ω |
Pin assignment
Raspberry Pi Pico | Sensor |
---|---|
GPIO26 | Signal |
3.3V | +V |
GND | GND |
GPIO18 | LED |
Code example
To load the following code example onto your Pico, we recommend using the Thonny IDE. All you have to do first is go to Run > Configure interpreter ... > Interpreter > Which kind of interpreter should Thonny use for running your code? and select MicroPython (Raspberry Pi Pico).
Now copy the code below into your IDE and click on Run.
# Load libraries
from machine import Pin, Timer
# Initialization of GPIO26 as input
# Initialization of GPIO18 as output
sensor = Pin(26, Pin.IN, Pin.PULL_DOWN)
LED = Pin(18, Pin.OUT, Pin.PULL_DOWN)
# Timer initialization
timer = Timer()
# Variable initialization
led_state = 0
# Function: LED is switched ON/OFF
def step(timer):
global led_state
if led_state == 0:
LED.value(1)
led_state = 1
else:
LED.value(0)
led_state = 0
# function so that the timer is triggered after the interrupt is triggered
# to execute the step()-function
def shake(pin):
# Set debounce function around timer
timer.init(mode=Timer.ONE_SHOT, period=100, callback=step)
# Initialization interrupt
sensor.irq(trigger=Pin.IRQ_FALLING, handler=shake)