KY-021 Mini reed magnet
If a magnetic field is detected, the two input pins are short-circuited by pulling them towards each other.
- Arduino
- Raspberry Pi
- Raspberry Pi Pico
- Micro:Bit
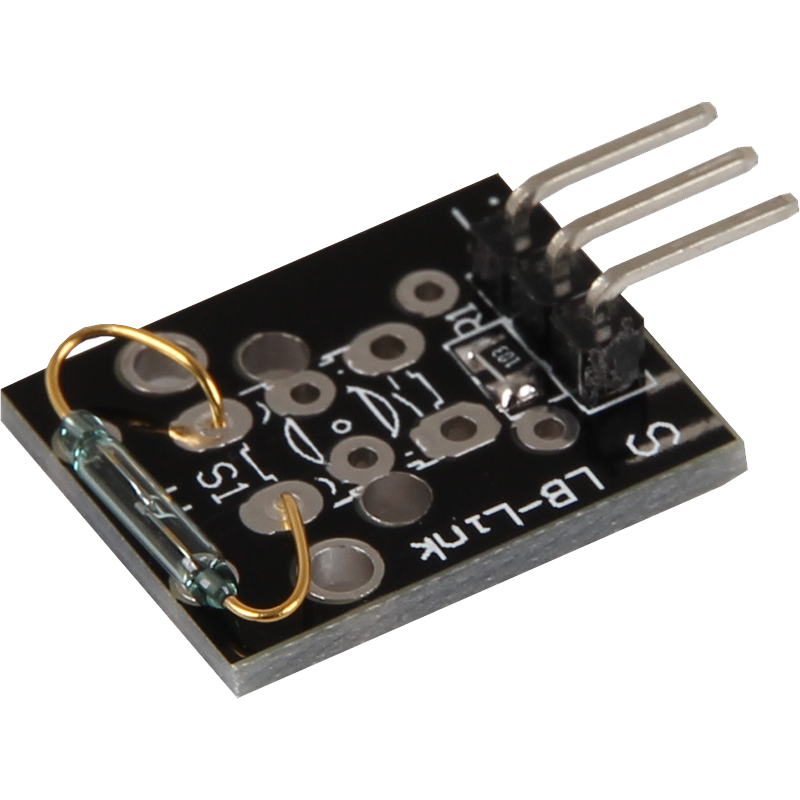
This module contains a mini reed magnet switch that short-circuits the two input pins when a magnetic field is detected. Inside the switch there are two contact tongues that attract each other in the presence of a magnetic field and thus close the circuit. As soon as a magnetic field is detected, the two input pins are connected together. This module is ideal for applications such as door and window alarms, position sensors or other projects where the presence of a magnet needs to be detected. It is easy to integrate and provides a reliable method of magnetic field detection.
Technical Data | |
---|---|
Operating voltage | 3,3 V - 5 V |
Dimensions | 19 x 18,5 x 9 mm |
Pin assignment
Arduino | Sensor |
---|---|
Pin 10 | signal |
5 V | +V |
GND | GND |
Code example
To load the following code example onto your Arduino, we recommend using the Arduino IDE. In the IDE, you can select the appropriate port and board for your device.
Copy the code below into your IDE. To upload the code to your Arduino, simply click on the upload button.
int reed_magnet = 10; // Declaration of the sensor input pin
int value; // Temporary variable
void setup () {
pinMode(reed_magnet, INPUT); // Initialization sensor pin
Serial.begin(9600); // Initialization of the serial monitor
Serial.println("KY-021 Magnetic field detection");
}
void loop () {
// The current signal at the sensor is read out
value = digitalRead(reed_magnet);
// If a signal could be detected, this is displayed on the serial monitor.
if (value == LOW) {
Serial.println("Magnetic field detected");
delay(100); // 100 ms break
}
}
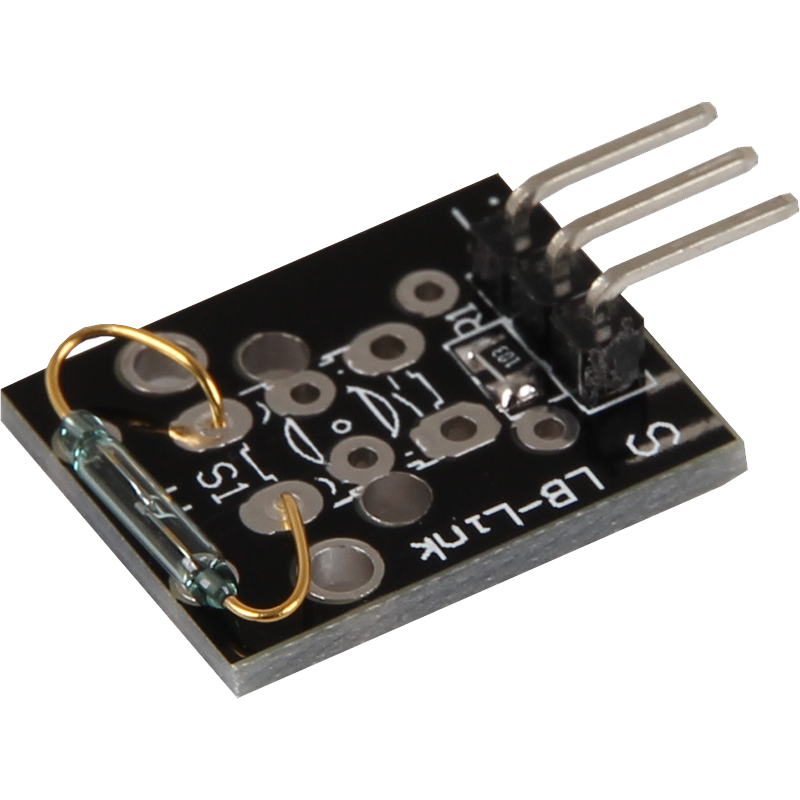
This module contains a mini reed magnet switch that short-circuits the two input pins when a magnetic field is detected. Inside the switch there are two contact tongues that attract each other in the presence of a magnetic field and thus close the circuit. As soon as a magnetic field is detected, the two input pins are connected together. This module is ideal for applications such as door and window alarms, position sensors or other projects where the presence of a magnet needs to be detected. It is easy to integrate and provides a reliable method of magnetic field detection.
Technical Data | |
---|---|
Operating voltage | 3,3 V - 5 V |
Dimensions | 19 x 18,5 x 9 mm |
Pin assignment
Raspberry Pi | Sensor |
---|---|
GPIO 24 [Pin 18] | Signal |
3.3 V [Pin 1] | +V |
GND [Pin 6] | GND |
Code example
This is an example program where a console output is generated as soon as a signal is detected.
from gpiozero import Button
import time
# The sensor is initialized as a button object with the internal pull-up resistor activated.
sensor = Button(24, pull_up=True)
print("Sensor test [press CTRL+C to end the test]")
# This function is executed when a signal is detected (falling edge).
def ausgabeFunktion():
print("Signal recognized")
# The 'outputFunction' function is bound to the 'when_pressed' event of the sensor.
sensor.when_pressed = ausgabeFunktion
# Main program loop
try:
while True:
time.sleep(1)
# Clean-up work after the program has been completed
except KeyboardInterrupt:
print("Program ended")
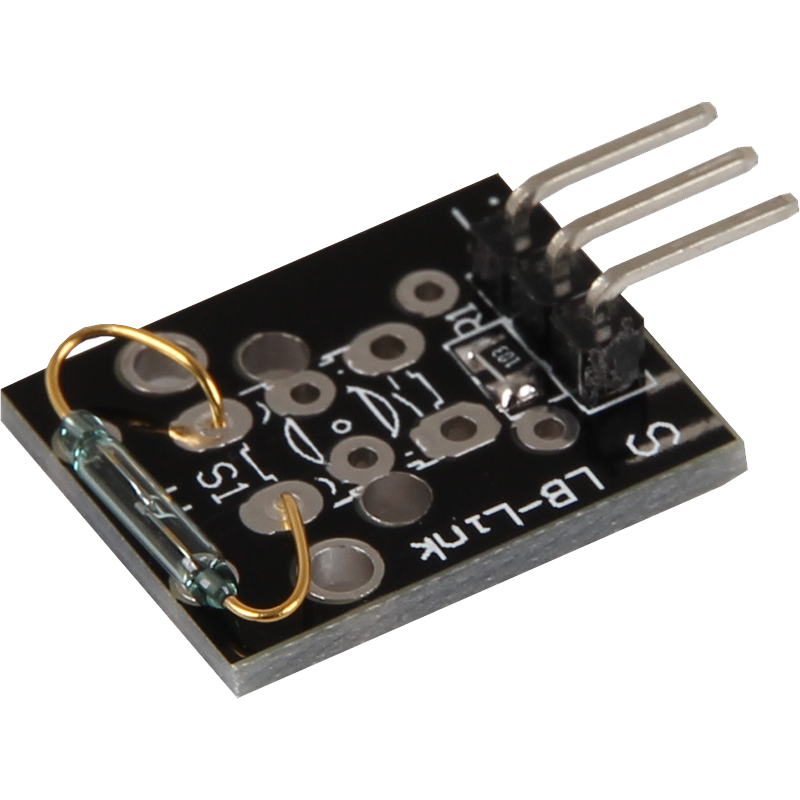
This module contains a mini reed magnet switch that short-circuits the two input pins when a magnetic field is detected. Inside the switch there are two contact tongues that attract each other in the presence of a magnetic field and thus close the circuit. As soon as a magnetic field is detected, the two input pins are connected together. This module is ideal for applications such as door and window alarms, position sensors or other projects where the presence of a magnet needs to be detected. It is easy to integrate and provides a reliable method of magnetic field detection.
Technical Data | |
---|---|
Operating voltage | 3,3 V - 5 V |
Dimensions | 19 x 18,5 x 9 mm |
Pin assignment
Micro:Bit | Sensor |
---|---|
Pin 1 | Signal |
3 V | +V |
GND | GND |
Code example
This is a sample program that outputs text serially when a signal is detected at the sensor.
pins.setPull(DigitalPin.P1, PinPullMode.PullUp)
basic.forever(function () {
serial.writeLine("" + (pins.digitalReadPin(DigitalPin.P1)))
if (pins.digitalReadPin(DigitalPin.P1) == 0) {
serial.writeLine("Magnetic field detected!")
} else {
serial.writeLine("No magnetic field detected")
}
serial.writeLine("_____________________________________")
basic.pause(1000)
})
Sample program download
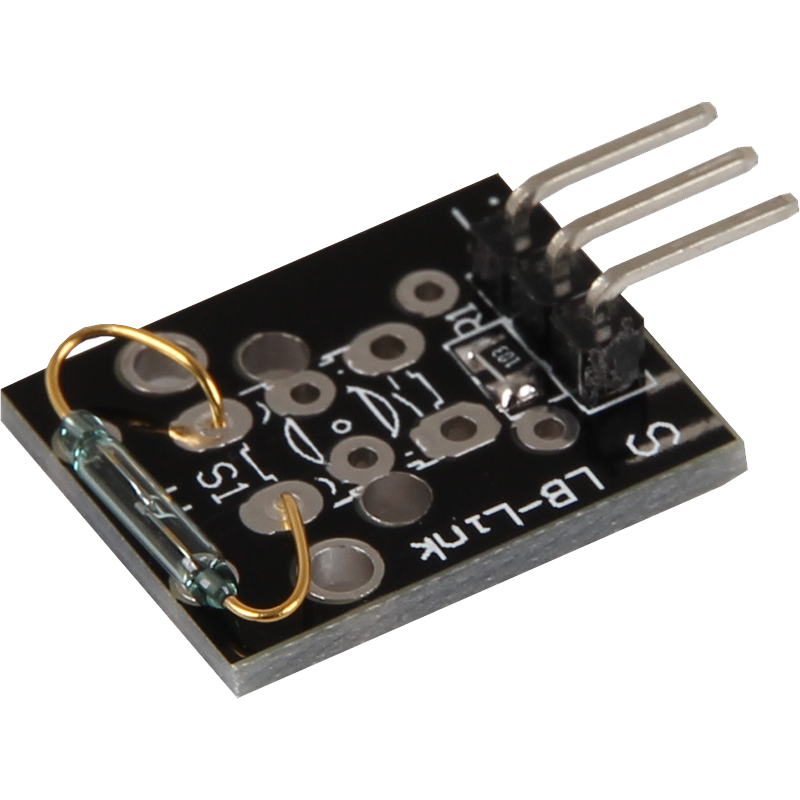
This module contains a mini reed magnet switch that short-circuits the two input pins when a magnetic field is detected. Inside the switch there are two contact tongues that attract each other in the presence of a magnetic field and thus close the circuit. As soon as a magnetic field is detected, the two input pins are connected together. This module is ideal for applications such as door and window alarms, position sensors or other projects where the presence of a magnet needs to be detected. It is easy to integrate and provides a reliable method of magnetic field detection.
Technical Data | |
---|---|
Operating voltage | 3,3 V - 5 V |
Dimensions | 19 x 18,5 x 9 mm |
Pin assignment
Raspberry Pi Pico | Sensor |
---|---|
GPIO26 | Signal |
3.3V | +V |
GND | GND |
Code example
This is a sample program that outputs text serially when a signal is detected at the sensor.
To load the following code example onto your Pico, we recommend using the Thonny IDE. All you have to do first is go to Run > Configure interpreter ... > Interpreter > Which kind of interpreter should Thonny use for running your code? and select MicroPython (Raspberry Pi Pico).
Now copy the code below into your IDE and click on Run.
# Load libraries
from machine import Pin, Timer
# Initialization of GPIO as input
sensor = Pin(26, Pin.IN, Pin.PULL_DOWN)
# Create timer
timer = Timer()
# Variables initialization
counter = 0
print("KY-021 Magnetic field detection")
# Function for counting steps
def step(timer):
print("Magnetic field detected")
# Function that is executed when a magnetic field is detected
def magnet(pin):
# Set debounce function timer
timer.init(mode=Timer.ONE_SHOT, period=500, callback=step)
# Initialization of the interrupt
sensor.irq(trigger=Pin.IRQ_FALLING, handler=magnet)