KY-031 Knock sensor
If the sensor is exposed to a knock/shock, the two output pins are short-circuited.
- Arduino
- Raspberry Pi
- Raspberry Pi Pico
- Micro:Bit
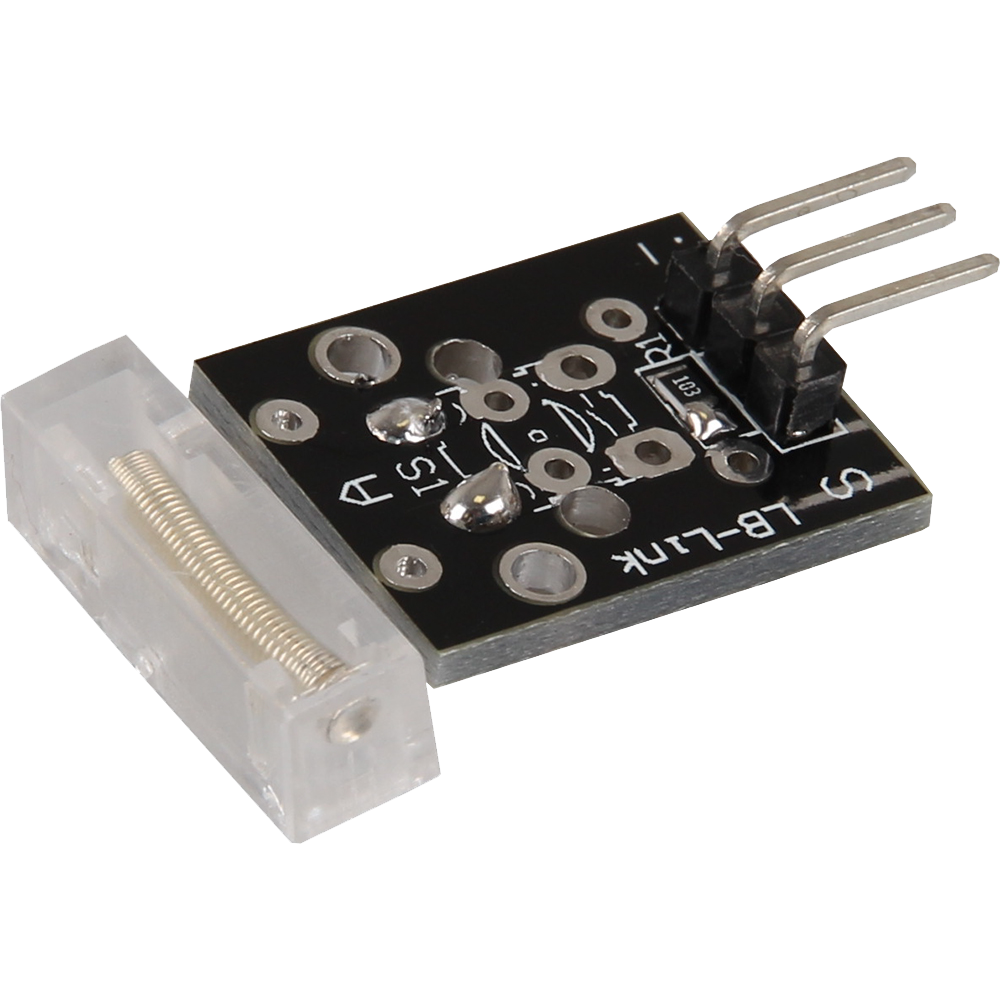
This module is a knock sensor. If the sensor is exposed to knocking or vibration, the two output pins are short-circuited. This reaction makes it possible to reliably detect vibrations or knocking events and pass them on as a signal. The knock sensor is ideal for projects where touch or vibration detection is required, such as in security systems, interactive devices or as an input method in various electronics projects. Its simple operation and reliability make it a versatile component for numerous applications.
Pin assignment
Arduino | Sensor |
---|---|
Pin 10 | Signal |
5 V | +V |
GND | GND |
Code example
To load the following code example onto your Arduino, we recommend using the Arduino IDE. In the IDE, you can select the appropriate port and board for your device.
Copy the code below into your IDE. To upload the code to your Arduino, simply click on the upload button.
int knock = 10; // Declaration of the sensor input pin
int value; // Temporary variable
void setup () {
pinMode(knock, INPUT); // Initialization sensor pin
Serial.begin(9600); // Initialization of the serial monitor
Serial.println("KY-031 Knock test");
}
void loop () {
// The current signal at the sensor is read out.
value = digitalRead(knock);
// If a signal could be detected, this is printed on the serial monitor.
if (value == LOW) {
Serial.println("Knock recognized");
delay(200); // 200 ms break
}
}
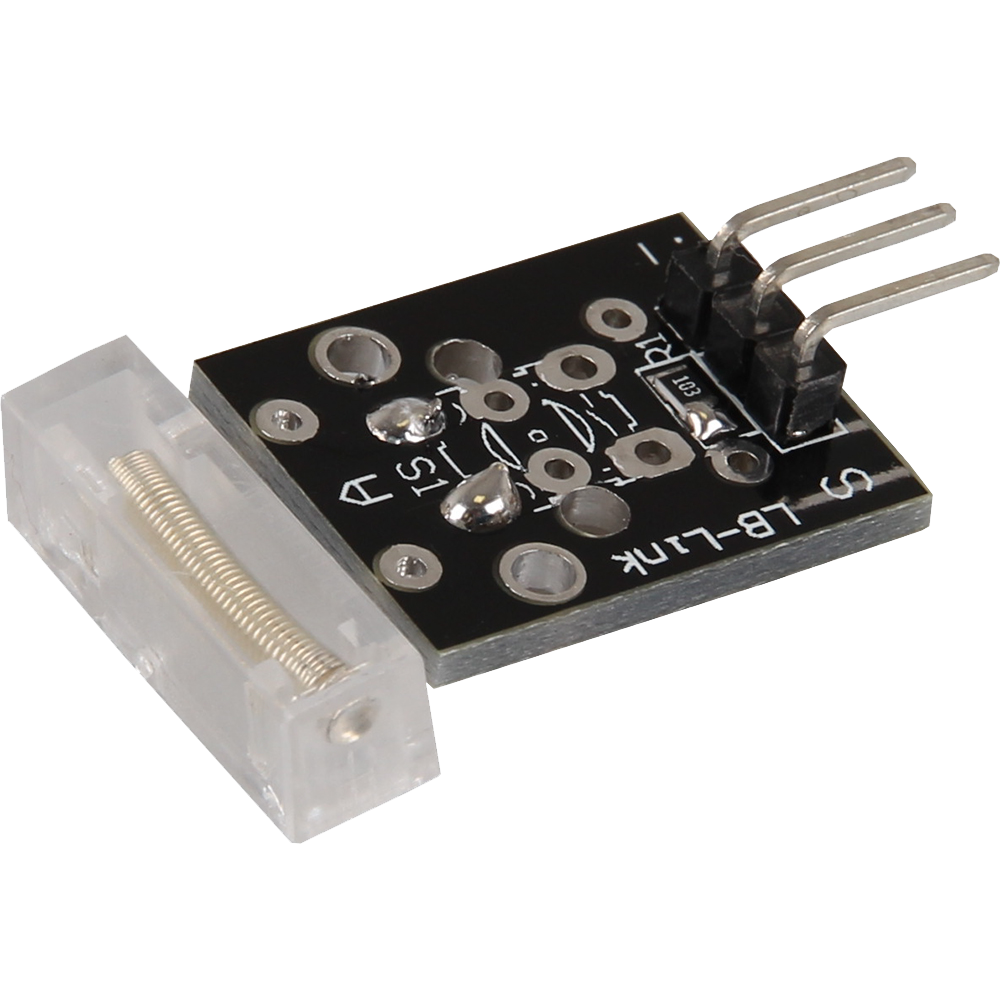
This module is a knock sensor. If the sensor is exposed to knocking or vibration, the two output pins are short-circuited. This reaction makes it possible to reliably detect vibrations or knocking events and pass them on as a signal. The knock sensor is ideal for projects where touch or vibration detection is required, such as in security systems, interactive devices or as an input method in various electronics projects. Its simple operation and reliability make it a versatile component for numerous applications.
Pin assignment
Raspberry Pi | Sensor |
---|---|
GPIO 24 [Pin 18] | Signal |
3.3 V [Pin 1 ] | +V |
GND [ Pin 6 ] | GND |
Code example
from gpiozero import DigitalInputDevice
import time
# Initialize the input pin to which the sensor is connected
sensor = DigitalInputDevice(24, pull_up=True) # Pull-up resistor is activated
print("Sensor test [press CTRL+C to end the test]”)
# This output function is executed when a signal is detected
def output_function():
print("Signal recognized")
# When a signal is detected (falling signal edge), the output function is triggered
sensor.when_activated = output_function
# Main program loop
try:
while True:
time.sleep(1)
# Refresh after the program has ended
except KeyboardInterrupt:
print("Program was interrupted by user")
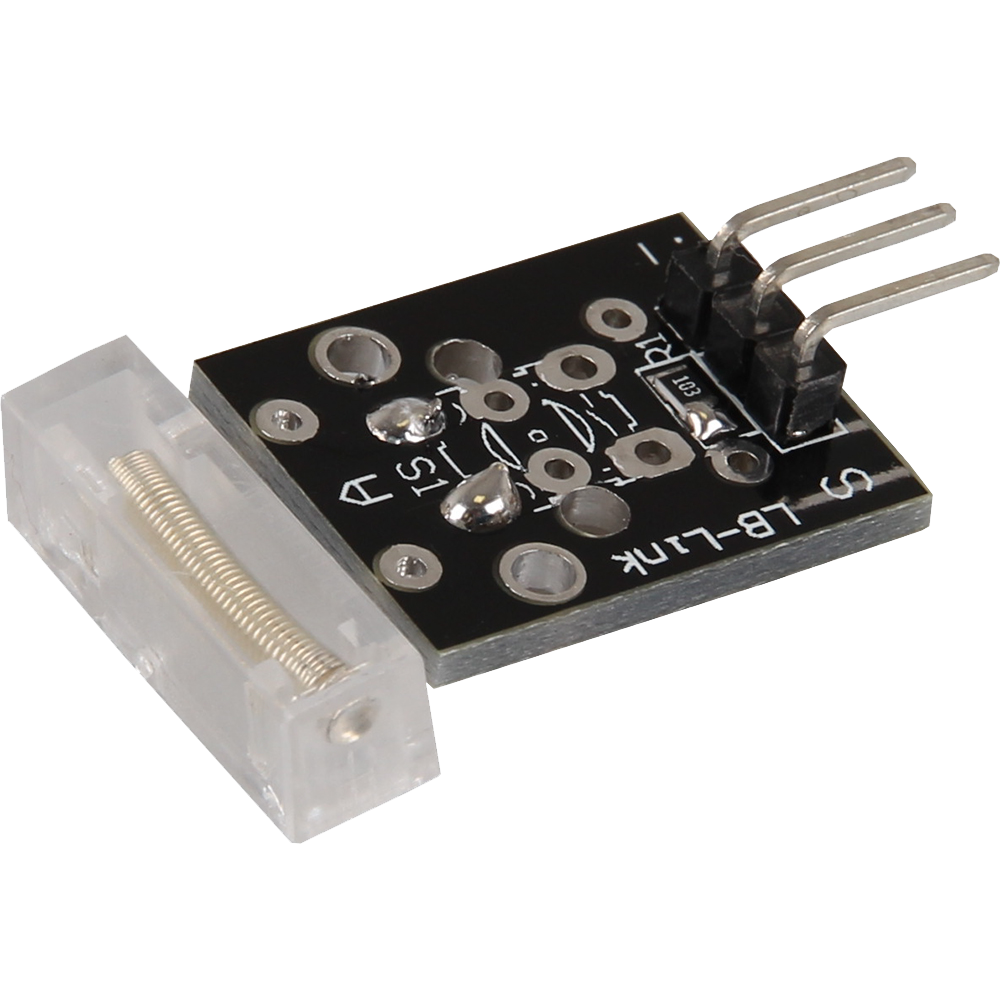
This module is a knock sensor. If the sensor is exposed to knocking or vibration, the two output pins are short-circuited. This reaction makes it possible to reliably detect vibrations or knocking events and pass them on as a signal. The knock sensor is ideal for projects where touch or vibration detection is required, such as in security systems, interactive devices or as an input method in various electronics projects. Its simple operation and reliability make it a versatile component for numerous applications.
Pin assignment
Micro: Bit | Sensor |
---|---|
Pin 1 | Signal |
3 V | +V |
GND | GND |
Code example
This is a sample program that outputs text serially when a signal is detected at the sensor.
pins.onPulsed(DigitalPin.P1, PulseValue.Low, function () {
serial.writeLine("Shock detected")
})
pins.setPull(DigitalPin.P1, PinPullMode.PullUp)
basic.forever(function () {
if (pins.digitalReadPin(DigitalPin.P1) == 1) {
serial.writeLine("No shock detected")
}
})
Sample program download
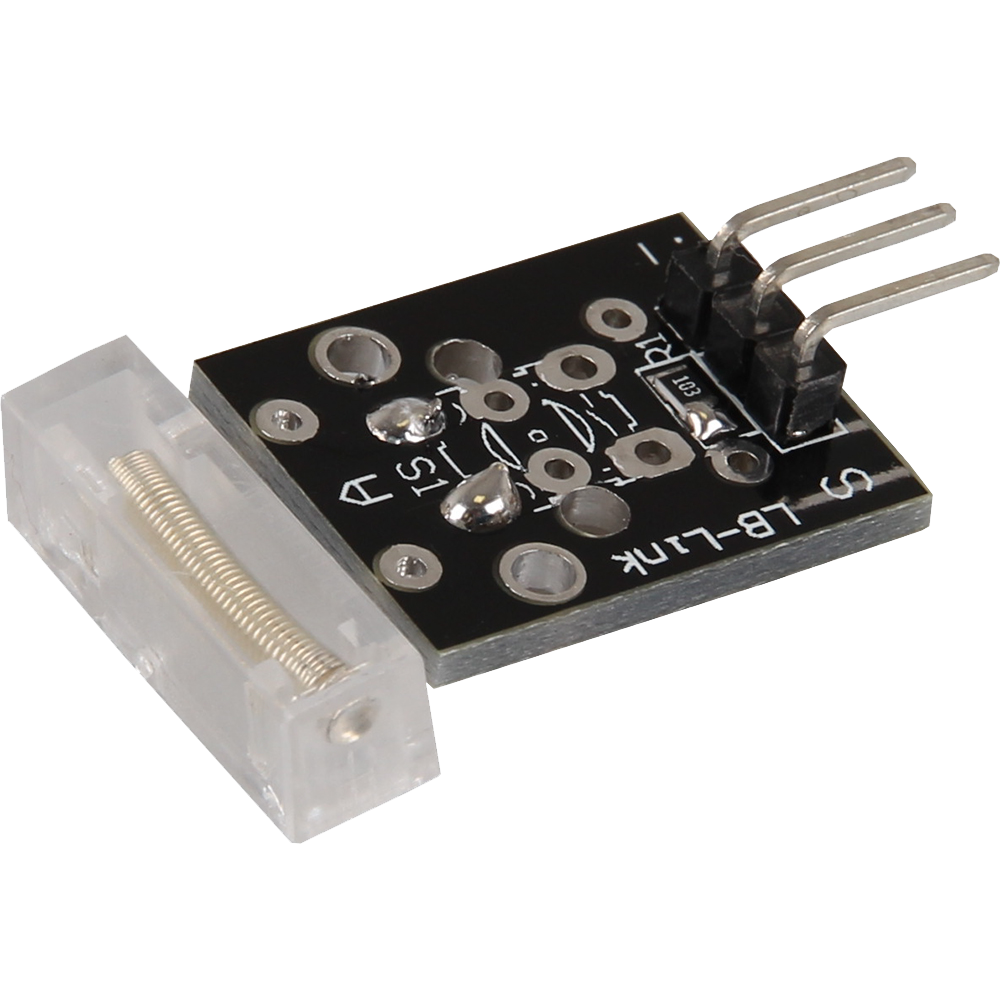
This module is a knock sensor. If the sensor is exposed to knocking or vibration, the two output pins are short-circuited. This reaction makes it possible to reliably detect vibrations or knocking events and pass them on as a signal. The knock sensor is ideal for projects where touch or vibration detection is required, such as in security systems, interactive devices or as an input method in various electronics projects. Its simple operation and reliability make it a versatile component for numerous applications.
Pin assignment
Raspberry Pi Pico | Sensor |
---|---|
GPIO18 | Signal |
3.3V | +V |
GND | GND |
Code example
This is a sample program that counts up and outputs text serially when a signal is detected at the sensor.
To load the following code example onto your Pico, we recommend using the Thonny IDE. All you have to do first is go to Run > Configure interpreter ... > Interpreter > Which kind of interpreter should Thonny use for running your code? and select MicroPython (Raspberry Pi Pico).
Now copy the code below into your IDE and click on Run.
# Load Libraries
from machine import Pin, Timer
# Initialization of GPIO as input
sensor = Pin(18, Pin.IN, Pin.PULL_DOWN)
# Create timer
timer = Timer()
# Variables initialization
counter = 0
print("KY-031 Vibration detection")
# Function for counting steps
def step(timer):
global counter
counter = counter + 1
print("Shock recognized:", counter)
# Function that is executed on vibration
def shake(pin):
# Set debounce function timer
timer.init(mode=Timer.ONE_SHOT, period=100, callback=step)
# Initialization of the interrupt
sensor.irq(trigger=Pin.IRQ_FALLING, handler=shake)