KY-033 Linetracking sensor
The sensor module detects whether there is a light-reflecting or light-absorbing surface in front of the sensor.
- Arduino
- Raspberry Pi
- Raspberry Pi Pico
- Micro:Bit
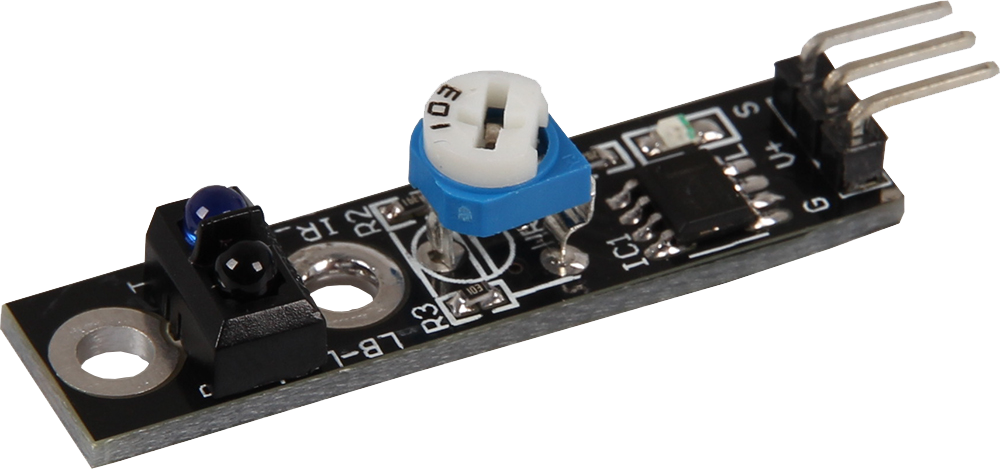
The sensor module detects whether there is a light-reflecting or light-absorbing surface in front of the sensor. This is output at the digital output of the sensor. This behavior can be used, for example, in controllers such as those used in robots for autonomous line tracking. The sensitivity of the sensor can additionally be adjusted via the two controllers.
State 1: Line tracker is over a line [LED on the module: Off] [Sensor Signal: Digital On].
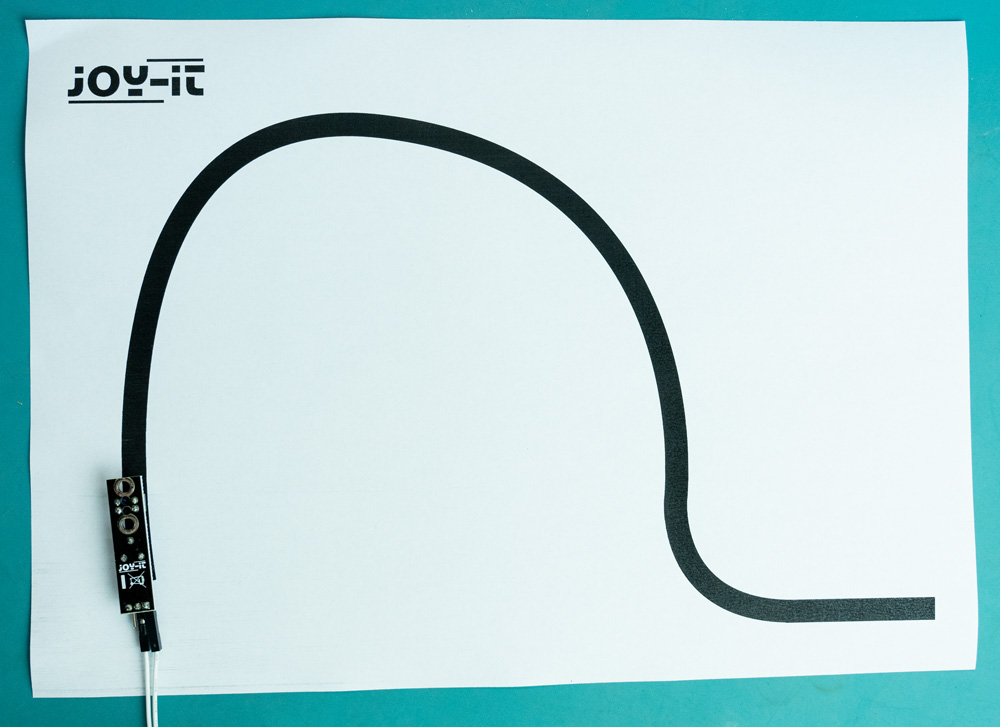
State 2: Line Tracker is outside a line [LED on the module: On] [Sensor Signal: Digital Off].
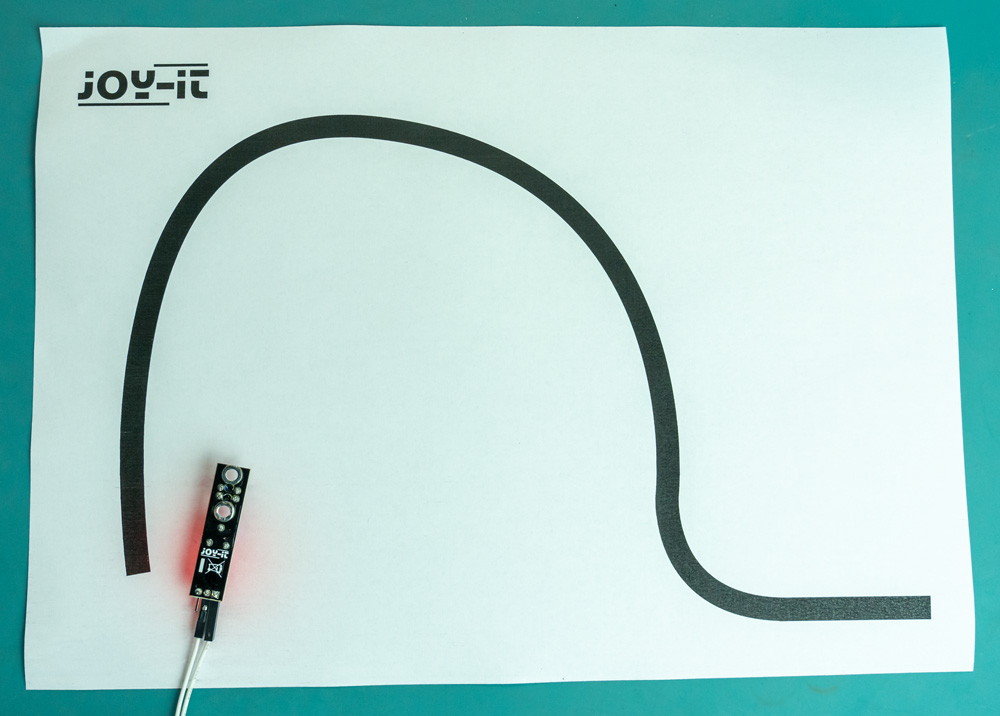
Pin assignment
Code example Arduino
Connection assignment Arduino
Arduino | Sensor |
---|---|
5 V | +V |
GND | GND |
Pin 10 | Signal |
The program reads the current state of the sensor pin and displays in the serial console whether the line tracker is currently on the line or not.
int Sensor = 10; // Declaration of the sensor input spin
void setup ( )
{
Serial.begin (9600) ; // Initialization serial output
pinMode (Sensor, INPUT) ; // Initialization Sensorpin
}
// The program reads the current state of the sensor pin and
// displays in the serial console whether the line tracker is currently
// on the line or not
void loop ( )
{
bool val = digitalRead (sensor) ; // The current signal on the sensor is read
if (val = = HIGH ) // If a signal has been detected, the LED is switched on.
{
Serial.println (“LineTracker is above the line”);
}
else
{
Serial.println (“Linetracker is out of line”);
}
Serial.println (“------------------------------------”) ;
delay (500) ; // Pasuse between the measurement of 500ms
}
Sample program Download
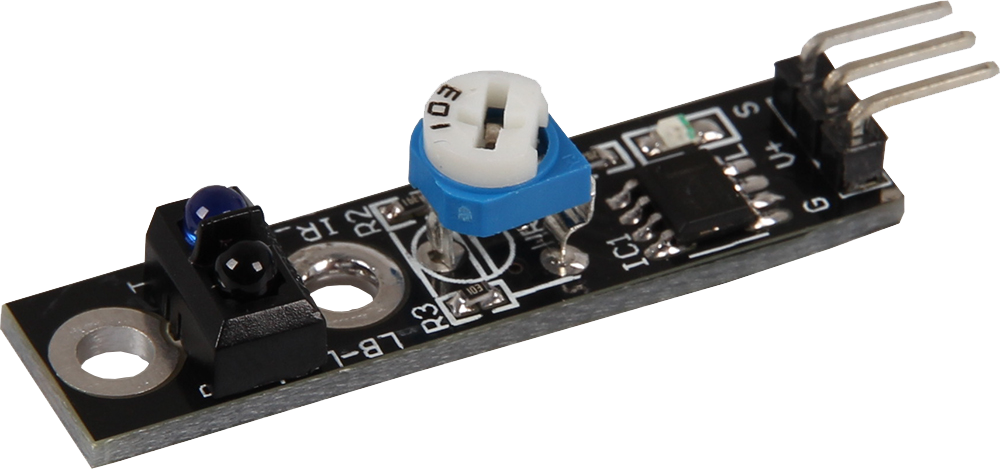
The sensor module detects whether there is a light-reflecting or light-absorbing surface in front of the sensor. This is output at the digital output of the sensor. This behavior can be used, for example, in controllers such as those used in robots for autonomous line tracking. The sensitivity of the sensor can additionally be adjusted via the two controllers.
State 1: Line tracker is over a line [LED on the module: Off] [Sensor Signal: Digital On].
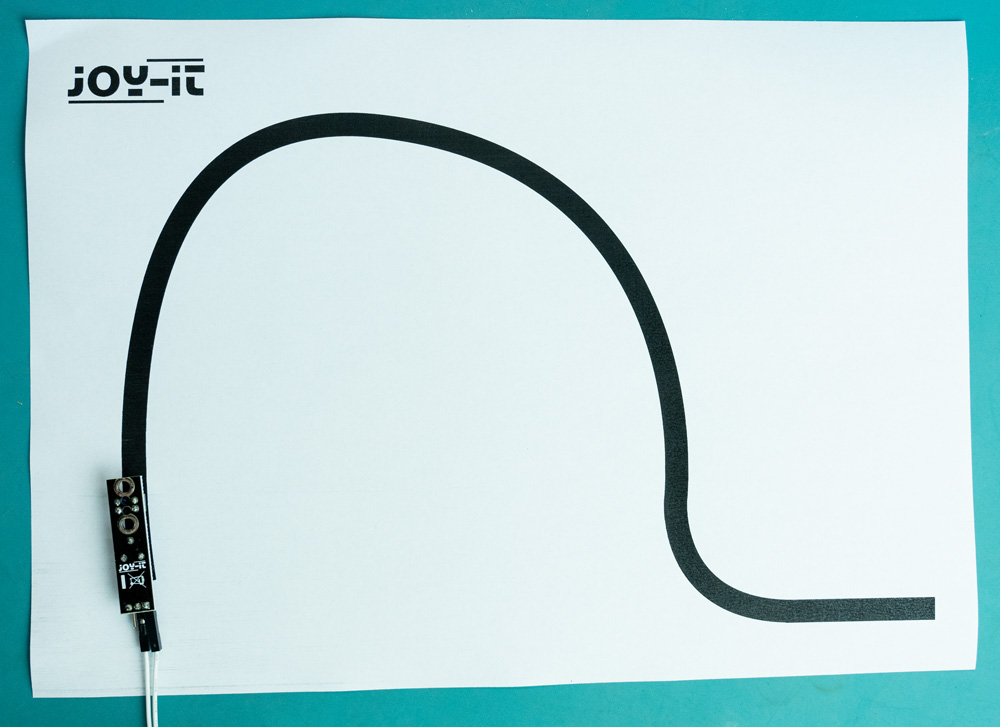
State 2: Line Tracker is outside a line [LED on the module: On] [Sensor Signal: Digital Off].
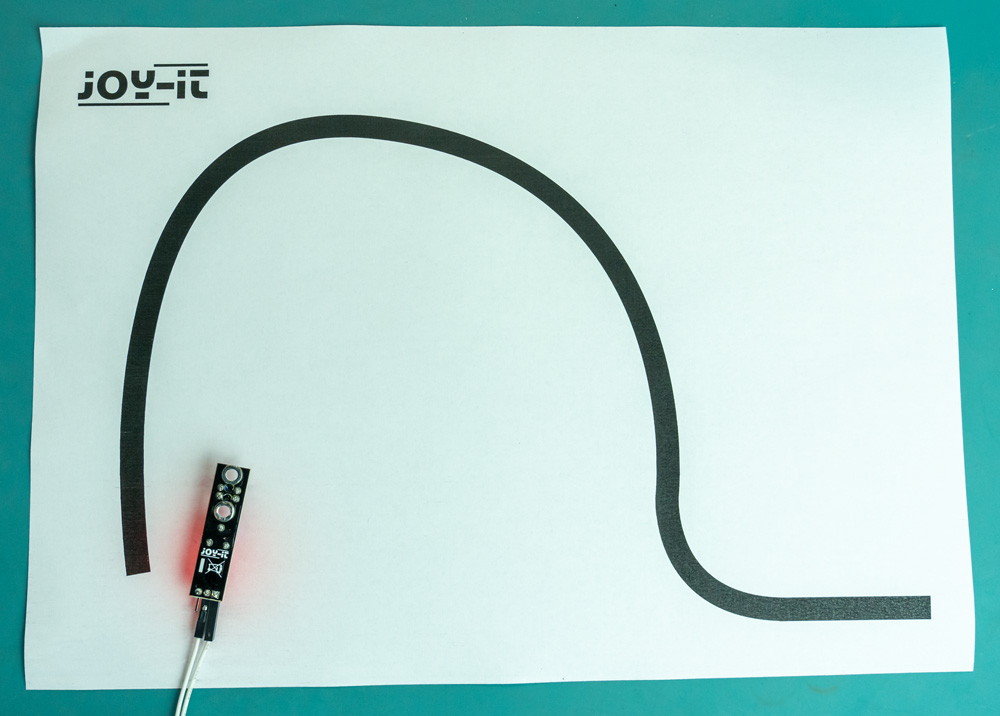
Pin assignment
Code example Raspberry Pi
Pin assignment Raspberry Pi
Raspberry Pi | Sensor |
---|---|
3,3 V [Pin 1] | +V |
GND [Pin 6] | GND |
GPIO 24 [Pin 18] | Signal |
The program reads the current state of the sensor pin and displays in the serial console whether the line tracker is currently on the line or not.
# Modules required are imported and set up
import RPi. GPIO as GPIO
import time
GPIO.setmode(GPIO.BCM)
# Here the input pin to which the sensor is connected is declared.
GPIO_PIN = 24
GPIO.setup (GPIO_PIN, GPIO.IN , pull_up_down = GPIO.PUD_UP)
# Pause between the output of the result is defined (in seconds)
delayTime = 0.5
print ("Sensor test [press CTRL+C to end the test]")
# Main program loop
try:
while True:
if GPIO.input(GPIO_PIN) == True:
print ("LineTracker is above the line")
else:
print ("Line tracker is out of line")
print (" ---------------------------------------")
# Reset + Delay
time.sleep(delayTime)
# Clean up after the program is finished
except KeyboardInterrupt:
GPIO.cleanup ()
Sample program Download
To start with the command:
sudo python3 KY033-RPi.py
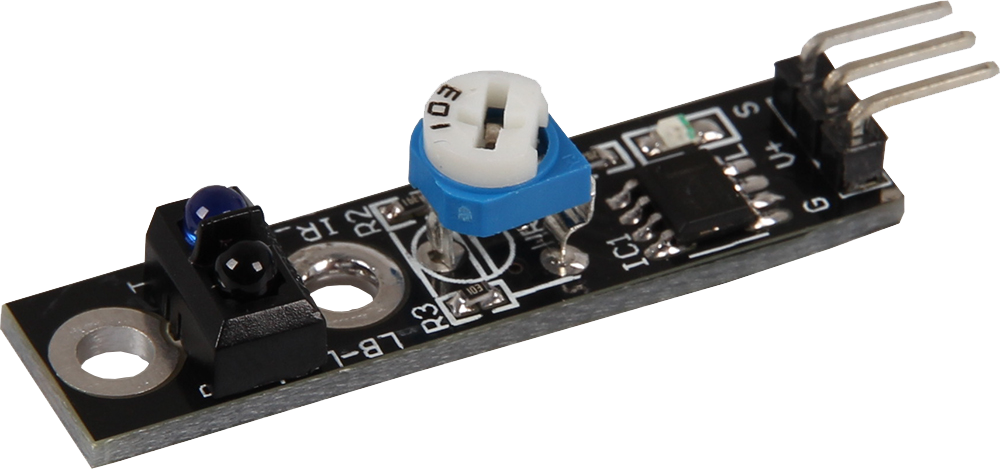
The sensor module detects whether there is a light-reflecting or light-absorbing surface in front of the sensor. This is output at the digital output of the sensor. This behavior can be used, for example, in controllers such as those used in robots for autonomous line tracking. The sensitivity of the sensor can additionally be adjusted via the two controllers.
State 1: Line tracker is over a line [LED on the module: Off] [Sensor Signal: Digital On].
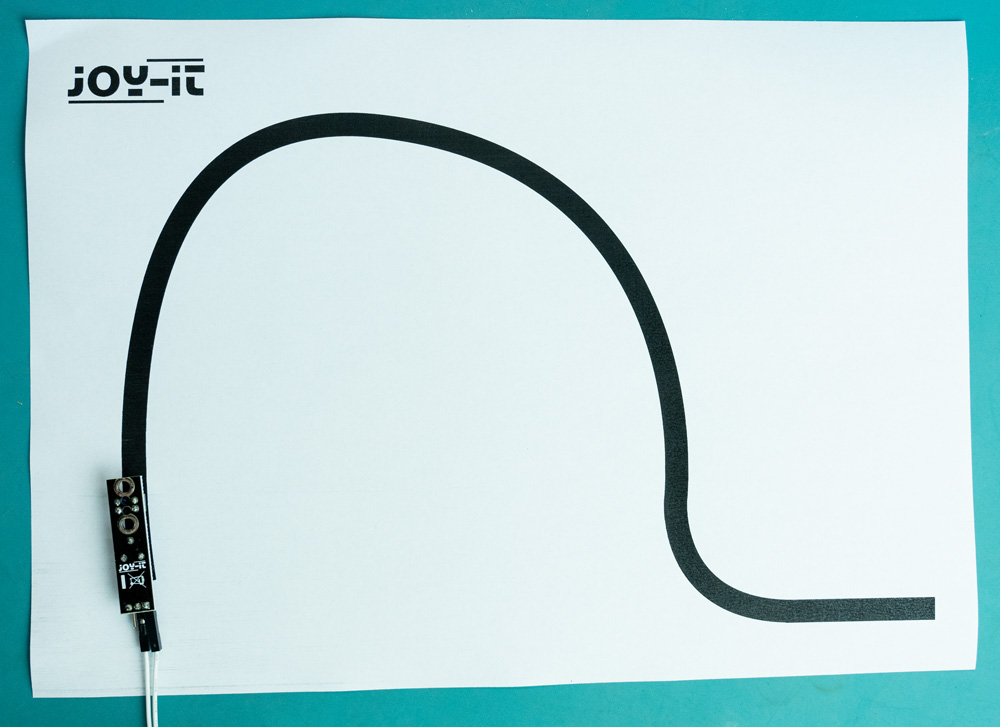
State 2: Line Tracker is outside a line [LED on the module: On] [Sensor Signal: Digital Off].
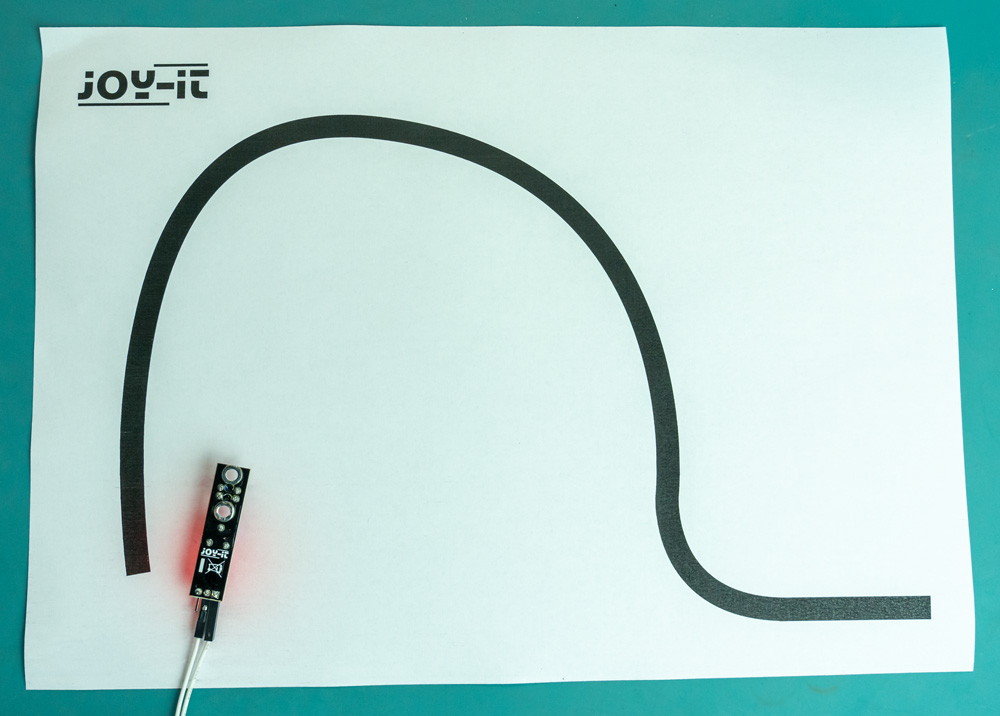
Pin assignment
Code example Micro:Bit
Pin assignment Micro : Bit:
Micro: Bit | Sensor |
---|---|
Pin 1 | Signal |
3 V | +V |
GND | GND |
This is a sample program that outputs text serially when a signal is detected at the sensor.
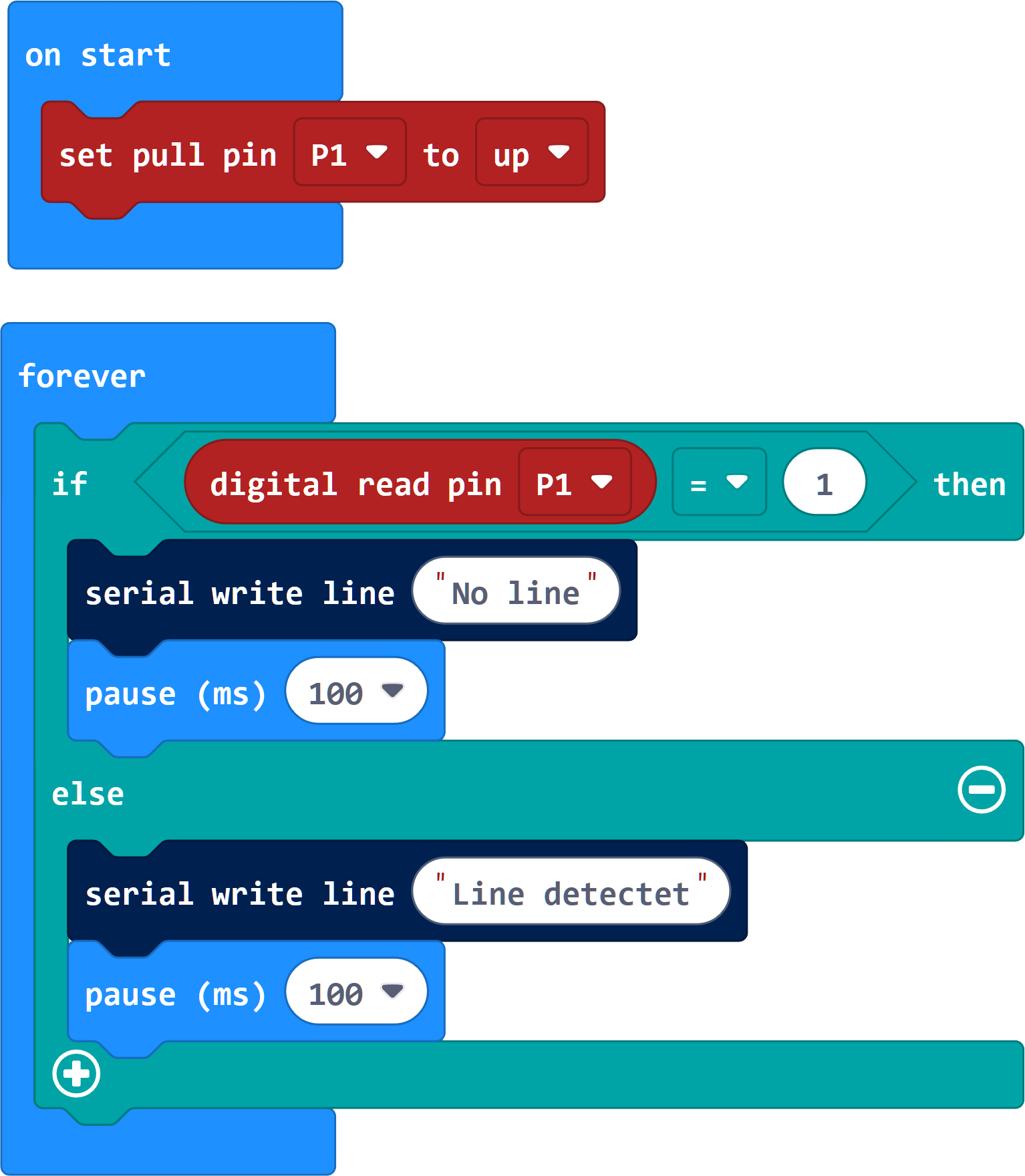
Sample program download
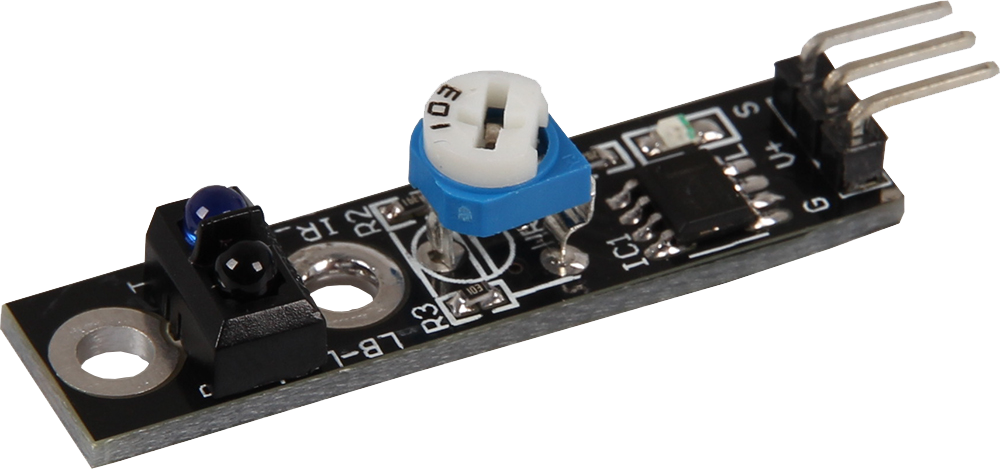
The sensor module detects whether there is a light-reflecting or light-absorbing surface in front of the sensor. This is output at the digital output of the sensor. This behavior can be used, for example, in controllers such as those used in robots for autonomous line tracking. The sensitivity of the sensor can additionally be adjusted via the two controllers.
State 1: Line tracker is over a line [LED on the module: Off] [Sensor Signal: Digital On].
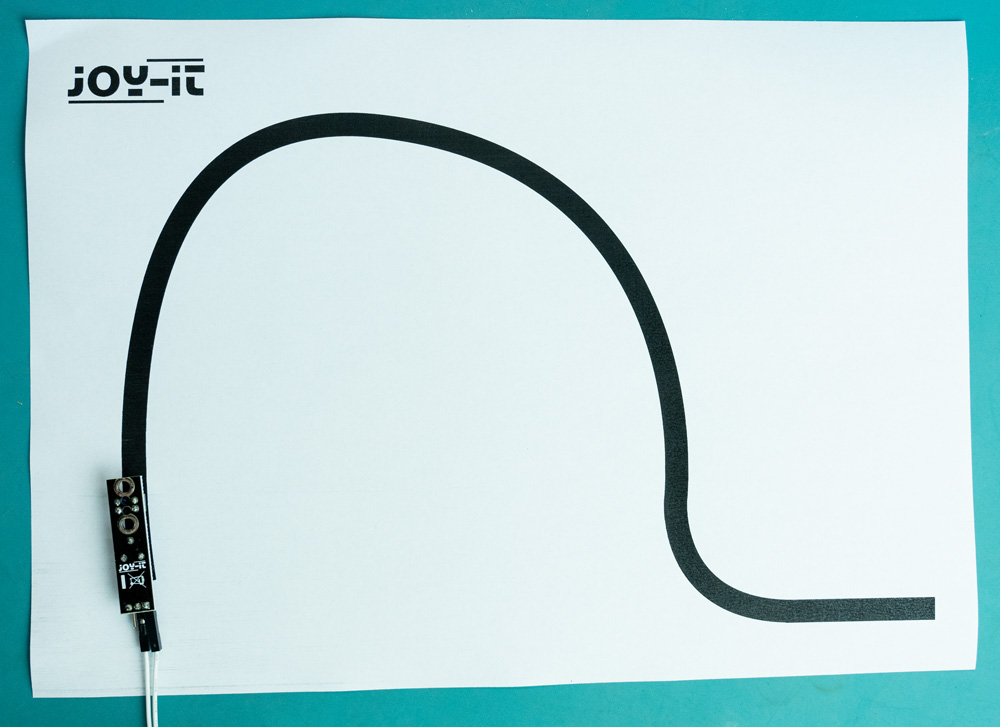
State 2: Line Tracker is outside a line [LED on the module: On] [Sensor Signal: Digital Off].
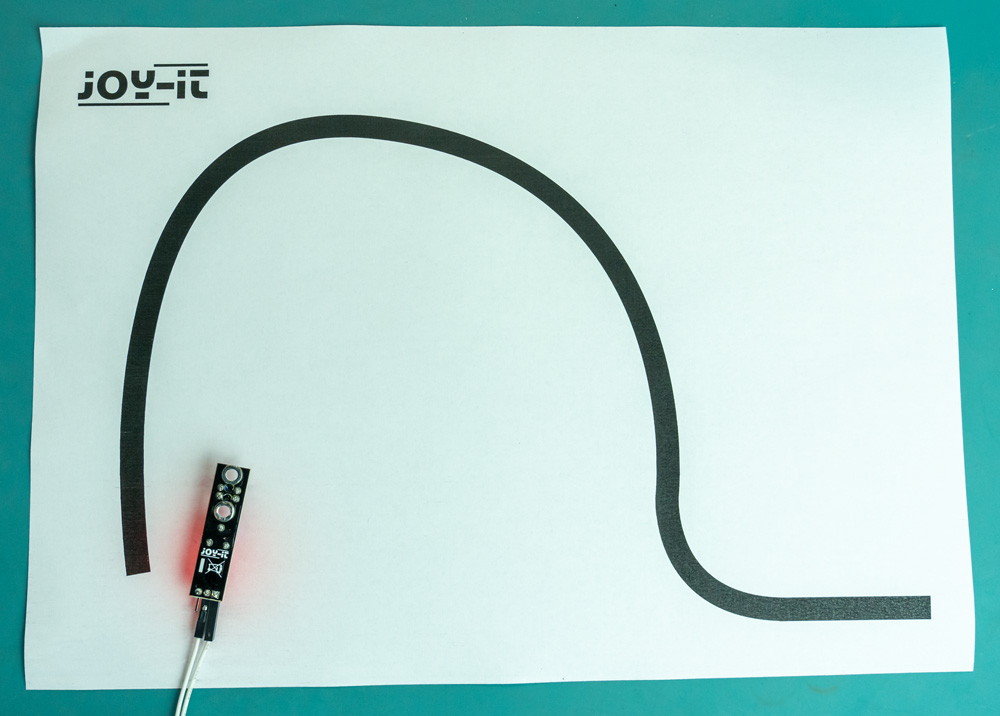
Pin assignment
Code example Raspbery Pi Pico
Pin assignment Raspbery Pi Pico
Raspbery Pi Pico | Sensor |
---|---|
3.3V | +V |
GND | GND |
GPIO18 | Signal |
The program reads the current status of the sensor pin and outputs it in the serial output. Thus it can be determined whether the line tracker is on a line or not.
# Load libraries
from machine import Pin
from time import sleep
# Initialization of GPIO18 as input
sensor = Pin(18, Pin.IN, Pin.PULL_DOWN)
# Continuous loop for continuous serial output
while True:
if sensor.value() == 0:
print("No line present")
else:
print("Line present")
print("------------------------------------------------------")
sleep(1)