KY-015 Combi-Sensor (temperature & humidity)
This sensor is a mixture of temperature sensor and humidity sensor in a compact design.
- Arduino
- Raspberry Pi
- Raspberry Pi Pico
- Micro:Bit
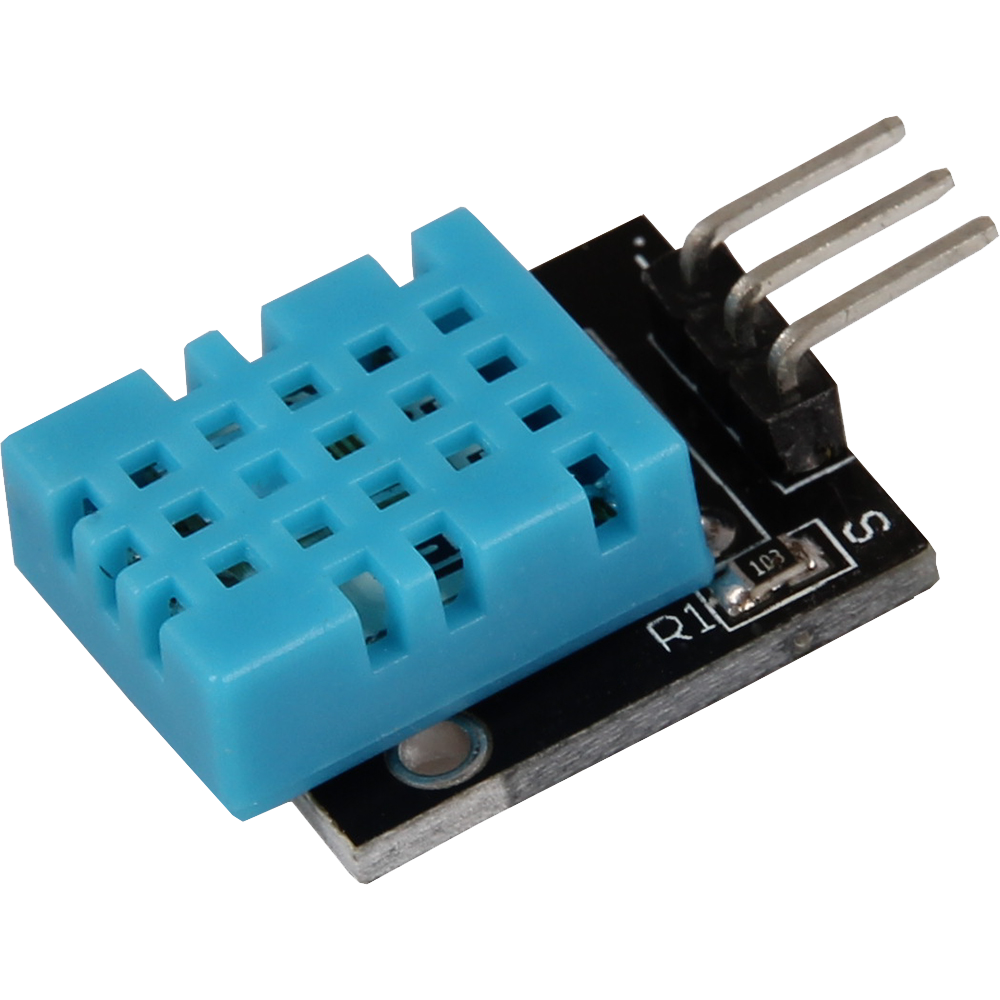
The DHT11 sensor is a combination of temperature and humidity sensor in a compact design. It measures temperatures from 0°C to 50°C with an accuracy of ±2°C and relative humidity from 20% to 90% with an accuracy of ±5%. Due to its low sampling rate, the sensor only provides new readings every two seconds, making it ideal for long-term measurements. The DHT11 is easy to integrate into microcontroller platforms such as Arduino and Raspberry Pi and requires no additional calibration thanks to factory calibration. Its robust design and reliability make it the optimal choice for continuous monitoring projects such as greenhouses, climate control systems and warehouse monitoring.
Technical Data | |
---|---|
Chipset | DHT11 |
Communication Protocol | 1-Wire |
Measuring range | 0 °C to 50 °C |
Measurement accuracy | ±2 °C |
Measurement Accuracy | ±5 % RH |
Measurable humidity | 20-90 % RH |
Pin assignment
Arduino | Sensor |
---|---|
Pin 2 | Signal |
5 V | +V |
GND | GND |
Code Example
To load the following code example onto your Arduino, we recommend using the Arduino IDE. In the IDE, you can select the appropriate port and the correct board for your device.
The following libraries are also used for the code example:
DHT-sensor-library by Adafruit | published under the MIT License.
Adafruit Unified Sensor by Adafruit | published under the Apache License 2.0
You can easily add these libraries via the library manager of the Arduino IDE. Then copy the code below into your IDE. To upload the code to the Arduino, simply click on the upload button.
This sensor does not output its measurement result as an analog signal to an output pin, but communicates it digitally encoded.
// Adafruit_DHT Library is inserted
#include <Adafruit_Sensor.h>
#include <DHT.h>
#include <DHT_U.h>
// The respective input pin can be declared here
#define DHTPIN 2
// The sensor is initialized
#define DHTTYPE DHT11 // DHT 11
DHT dht(DHTPIN, DHTTYPE);
void setup() {
Serial.begin(9600);
Serial.println("KY-015 Test - Temperature and humidity test:");
// Measurement is started
dht.begin();
}
// Main program loop
// The program starts the measurement and reads out the measured values
// A pause of 2 seconds is inserted between the measurements,
// so that a new measurement can be recorded during the next run.
void loop() {
// Two seconds pause between measurements
delay(2000);
// Humidity is measured
float h = dht.readHumidity();
// Temperature is measured
float t = dht.readTemperature();
// Checks whether the measurements have run through without errors
// If an error is detected, an error message is output here
if (isnan(h) || isnan(t)) {
Serial.println("Error when reading out the sensor");
return;
}
// Output to the serial console
Serial.println("-----------------------------------------------------------");
Serial.print(" Humidity: ");
Serial.print(h);
Serial.print(" %\t");
Serial.print(" Temperature: ");
Serial.print(t);
Serial.println(" °C ");
Serial.println("-----------------------------------------------------------");
Serial.println(" ");
}
Please note that the sensor provides a new measurement result only about every 2 seconds.
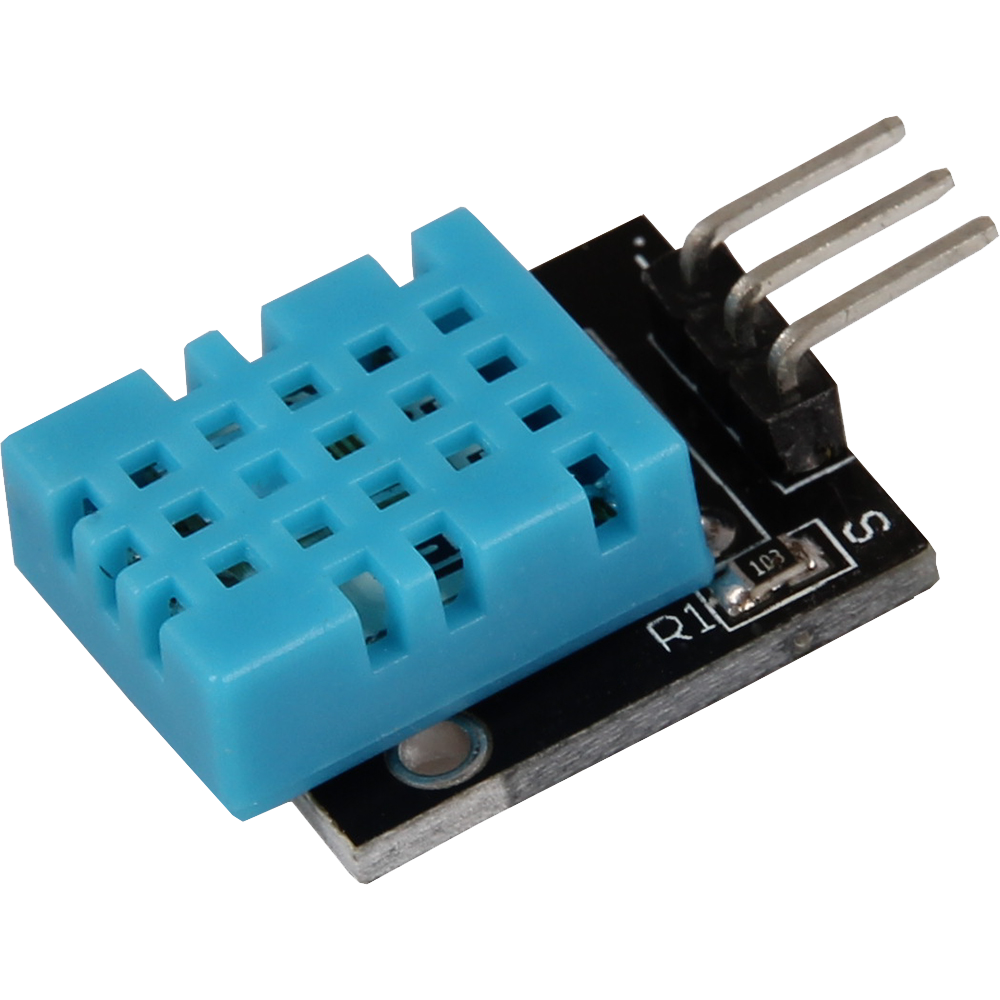
The DHT11 sensor is a combination of temperature and humidity sensor in a compact design. It measures temperatures from 0°C to 50°C with an accuracy of ±2°C and relative humidity from 20% to 90% with an accuracy of ±5%. Due to its low sampling rate, the sensor only provides new readings every two seconds, making it ideal for long-term measurements. The DHT11 is easy to integrate into microcontroller platforms such as Arduino and Raspberry Pi and requires no additional calibration thanks to factory calibration. Its robust design and reliability make it the optimal choice for continuous monitoring projects such as greenhouses, climate control systems and warehouse monitoring.
Technical Data | |
---|---|
Chipset | DHT11 |
Communication Protocol | 1-Wire |
Measuring range | 0 °C to 50 °C |
Measurement accuracy | ±2 °C |
Measurement Accuracy | ±5 % RH |
Measurable humidity | 20-90 % RH |
Pin assignment
Raspberry Pi | Sensor |
---|---|
GPIO 23 [Pin 16] | Signal |
+3.3 V [Pin 1] | +V |
GND [Pin 6] | GND |
Code Example
The program uses the corresponding Adafruit_CircuitPython_DHT library from Adafruit to control the DHT11 sensor, which is installed on this sensor module. This was released with the MIT OpenSource license.
This must be installed first. To do this, execute the following commands:
sudo apt-get update
sudo apt-get install build-essential python-dev
sudo apt install gpiod
sudo pip3 install python3-pip
This must be installed first. To do this, execute the following commands:
mkdir dein_projekt
cd dein_projekt
python -m venv --system-site-packages env
source env/bin/activate
Now install the library with the following command:
pip3 install adafruit-circuitpython-dht
After you have downloaded the library, you only need to enter the following command...
nano KY015-RPi.py
...create a new file on your Raspberry Pi and then copy the following code into the file you have just created.
import time
import board
import adafruit_dht
# Initialize the dht device with the data pin connected to pin 16 (GPIO 23) of the Raspberry Pi:
dhtDevice = adafruit_dht.DHT11(board.D23)
# You can pass DHT22 use_pulseio=False if you do not want to use pulseio.
# This may be necessary on a Linux single board computer like the Raspberry Pi,
# but it will not work in CircuitPython.
# dhtDevice = adafruit_dht.DHT22(board.D18, use_pulseio=False)
while True:
try:
# Print the values via the serial interface
temperature_c = dhtDevice.temperature
temperature_f = temperature_c * (9 / 5) + 32
humidity = dhtDevice.humidity
print("Temp: {:.1f} F / {:.1f} C Humidity: {}% ".format(temperature_f, temperature_c, humidity))
except RuntimeError as error:
# Errors happen quite often, DHT's are hard to read, just move on
print(error.args[0])
time.sleep(2.0)
continue
except Exception as error:
dhtDevice.exit()
raise error
time.sleep(2.0)
Please note that the sensor provides a new measurement result only about every 2 seconds.
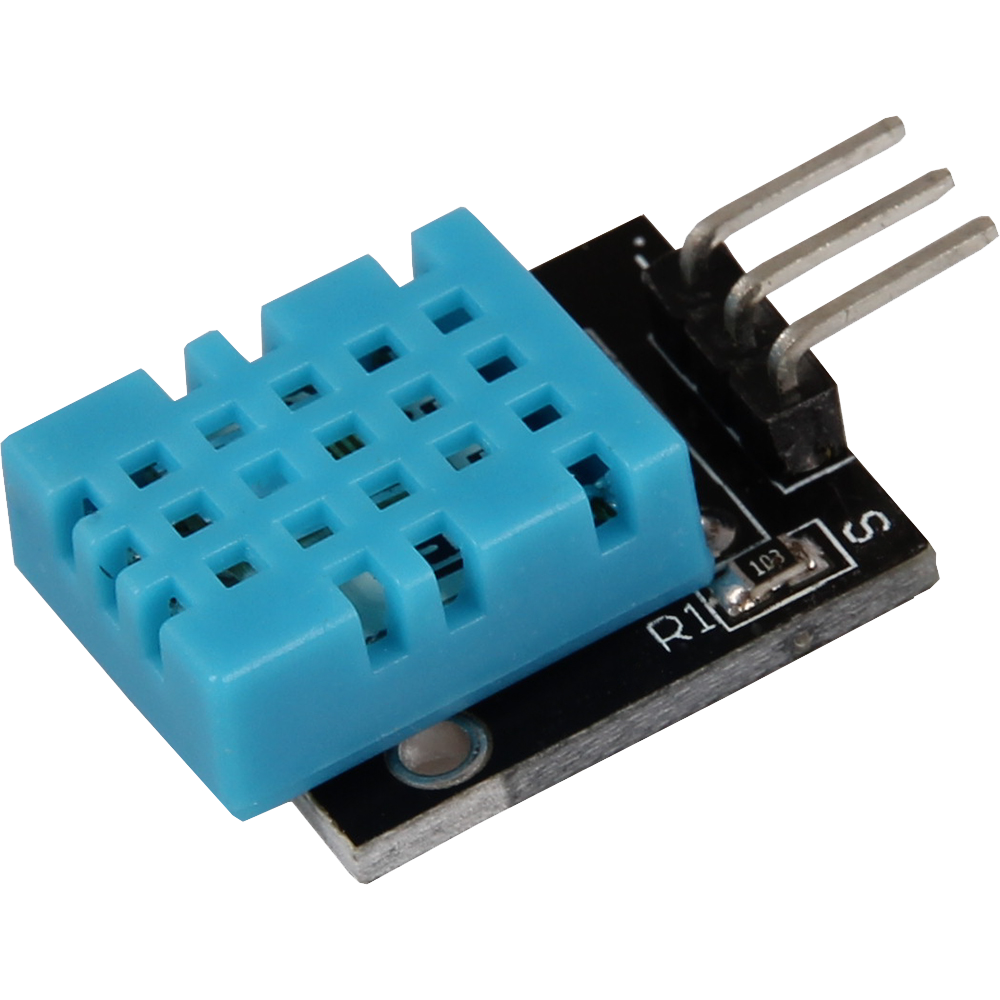
The DHT11 sensor is a combination of temperature and humidity sensor in a compact design. It measures temperatures from 0°C to 50°C with an accuracy of ±2°C and relative humidity from 20% to 90% with an accuracy of ±5%. Due to its low sampling rate, the sensor only provides new readings every two seconds, making it ideal for long-term measurements. The DHT11 is easy to integrate into microcontroller platforms such as Arduino and Raspberry Pi and requires no additional calibration thanks to factory calibration. Its robust design and reliability make it the optimal choice for continuous monitoring projects such as greenhouses, climate control systems and warehouse monitoring.
Technical Data | |
---|---|
Chipset | DHT11 |
Communication Protocol | 1-Wire |
Measuring range | 0 °C to 50 °C |
Measurement accuracy | ±2 °C |
Measurement Accuracy | ±5 % RH |
Measurable humidity | 20-90 % RH |
Pin assignment
Micro:Bit | Sensor |
---|---|
Pin 1 | Signal |
3 V | +V |
GND | GND |
Code Example
An additional library is needed for the following code example:
pxt-DHT11_DHT22 from alankrantas | published under the MIT License.
You must add this library to your IDE before using the code. Add the library to your IDE by clicking Extensions and typing the following URL in the search box: https://github.com/alankrantas/pxt-DHT11_DHT22.git. Bestätigen Sie die Suche mit [Enter].
basic.forever(function () {
dht11_dht22.queryData(
DHTtype.DHT11,
DigitalPin.P1,
true,
false,
true
)
basic.showString("T:" + dht11_dht22.readData(dataType.temperature) + "C")
basic.pause(2000)
dht11_dht22.queryData(
DHTtype.DHT11,
DigitalPin.P1,
true,
false,
true
)
basic.showString("H:" + dht11_dht22.readData(dataType.humidity) + "%")
basic.pause(2000)
})
Sample program download
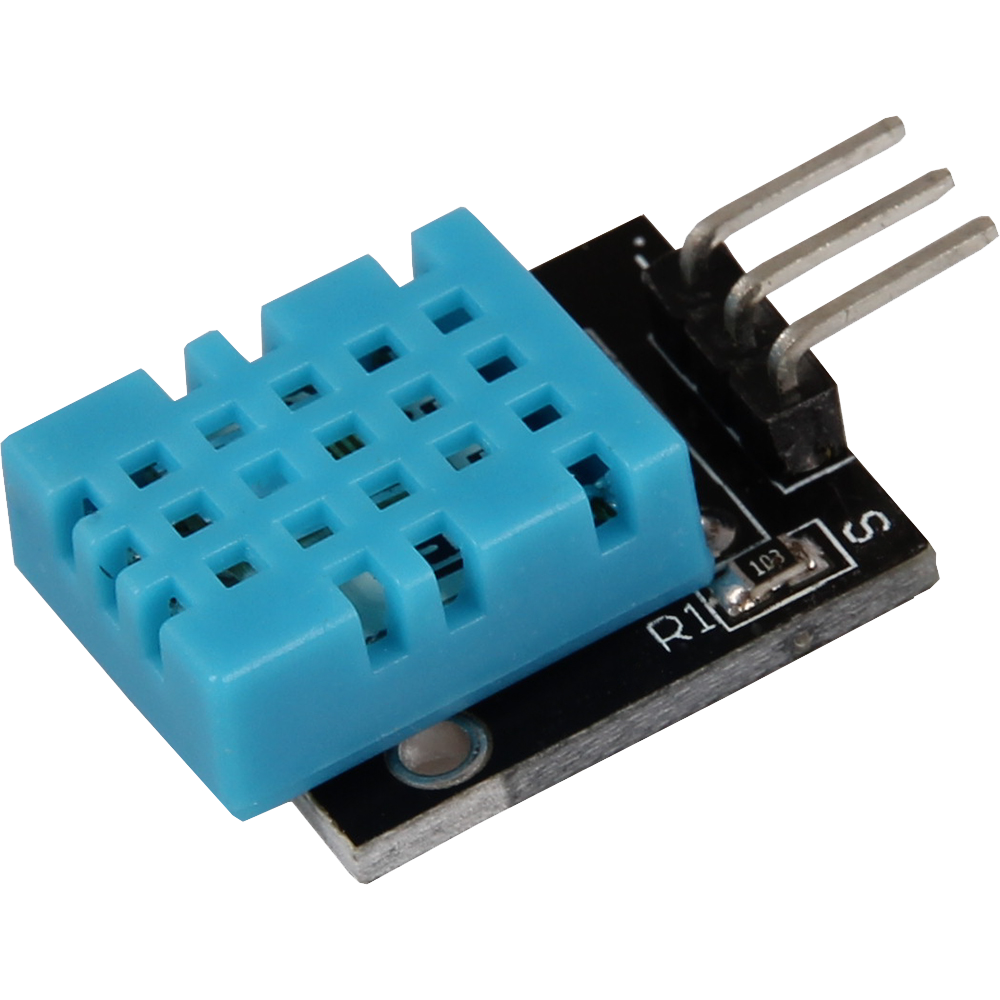
The DHT11 sensor is a combination of temperature and humidity sensor in a compact design. It measures temperatures from 0°C to 50°C with an accuracy of ±2°C and relative humidity from 20% to 90% with an accuracy of ±5%. Due to its low sampling rate, the sensor only provides new readings every two seconds, making it ideal for long-term measurements. The DHT11 is easy to integrate into microcontroller platforms such as Arduino and Raspberry Pi and requires no additional calibration thanks to factory calibration. Its robust design and reliability make it the optimal choice for continuous monitoring projects such as greenhouses, climate control systems and warehouse monitoring.
Technical Data | |
---|---|
Chipset | DHT11 |
Communication Protocol | 1-Wire |
Measuring range | 0 °C to 50 °C |
Measurement accuracy | ±2 °C |
Measurement Accuracy | ±5 % RH |
Measurable humidity | 20-90 % RH |
Pin assignment
Raspberry Pi Pico | Sensor |
---|---|
GPIO14 | Signal |
3.3V | +V |
GND | GND |
Code Example
This sensor does not output its measurement result as an analog signal on an output pin, but communicates it digitally encoded.
To load the following code example onto your Pico, we recommend using the Thonny IDE. All you have to do first is go to Run > Configure interpreter ... > Interpreter > Which kind of interpreter should Thonny use for running your code? and select MicroPython (Raspberry Pi Pico).
Now copy the code below into your IDE and click on Run.
# Load libraries
from machine import Pin
from utime import sleep
from dht import DHT11
# Initialization GPIO14 and DHT11
dht11_sensor = DHT11(Pin(14, Pin.IN, Pin.PULL_UP))
# Repeat (endless loop)
while True:
# Perform measurement
dht11_sensor.measure()
# Read values
temp = dht11_sensor.temperature()
humi = dht11_sensor.humidity()
# Output values
print("Temperature: {}°C Humidity: {:.0f}% ".format(temp, humi))
print()
sleep(2)
Please note that the sensor provides a new measurement result only about every 2 seconds.